Please use following steps given by spring and azure community to deploy spring boot app on azure:
1) Go inside of your app folder where you have pom file and run
make sure following plugins should be in pom file
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.springframework</groupId>
<artifactId>gs-spring-boot</artifactId>
<version>0.1.0</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.6.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- tag::actuator[] -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<!-- end::actuator[] -->
<!-- tag::tests[] -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- end::tests[] -->
</dependencies>
<properties>
<java.version>1.8</java.version>
<maven.build.timestamp.format>yyyyMMddHHmmssSSS</maven.build.timestamp.format>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<artifactId>maven-failsafe-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>com.microsoft.azure</groupId>
<artifactId>azure-webapp-maven-plugin</artifactId>
<version>0.1.5</version>
<configuration>
<authentication>
<serverId>azure-auth</serverId>
</authentication>
<resourceGroup>maven-plugin</resourceGroup>
<appName>maven-web-app-${maven.build.timestamp}</appName>
<region>westus</region>
<javaVersion>1.8</javaVersion>
<deploymentType>ftp</deploymentType>
<stopAppDuringDeployment>true</stopAppDuringDeployment>
<resources>
<resource>
<directory>${project.basedir}/target</directory>
<targetPath>/</targetPath>
<includes>
<include>*.jar</include>
</includes>
</resource>
<resource>
<directory>${project.basedir}</directory>
<targetPath>/</targetPath>
<includes>
<include>web.config</include>
</includes>
</resource>
</resources>
</configuration>
</plugin>
</plugins>
</build>
</project>
Note : Make sure you have created web app on azure with same name as
maven-web-app-${maven.build.timestamp}
Now create file on root with name "web.config" and add your jar in web.comfig
<?xml version="1.0" encoding="UTF-8"?>
<configuration>
<system.webServer>
<handlers>
<add name="httpPlatformHandler" path="*" verb="*" modules="httpPlatformHandler" resourceType="Unspecified"/>
</handlers>
<httpPlatform processPath="%JAVA_HOME%\bin\java.exe"
arguments="-Djava.net.preferIPv4Stack=true -Dserver.port=%HTTP_PLATFORM_PORT% -jar "%HOME%\site\wwwroot\azure-rest-example-app-0.1.0.jar"">
</httpPlatform>
</system.webServer>
</configuration>
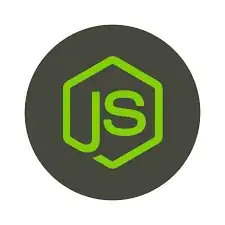
Now open azure CLI and run following commands
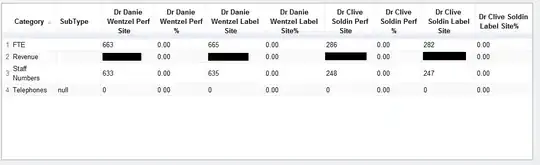
- mvn clean package
- mvn spring-boot:run
Make sure app is working fine on local.
Now use following commands if you have multiple account associated with your id
Now you need to create "Service Principals in Microsoft Azure"
1) Open a terminal window.
2) Sign into your Azure account with the Azure CLI by typing az login
3) Create an Azure service principal by typing az ad sp create-for-rbac --name "vaquarkhan" --password "yourpassword" (vaquarkhan is the user name and yourpassword is the password for the service principal).
az ad sp create-for-rbac --name "app-name" --password "password"
NOTE :if you getting error need to change settings---> here
"azure.graphrbac.models.graph_error.GraphErrorException: Guest users
are not allowed to perform this action."
if success
Azure should print out a JSON response resembling this:
{
"appId": "XXX-XXXX-XXX-XXX-XXXX",
"displayName": "vaquarkhan",
"name": "http://vaquarkhan",
"password": "yourpassword",
"tenant": "YYY-YYYY-YYY-YYY-YYYY"
}
Configure Maven to use your Azure service principal
1) Open your Maven settings.xml file in a text editor (usually found at either /etc/maven/settings.xml or $HOME/.m2/settings.xml).
<?xml version="1.0" encoding="UTF-8"?>
<settings xmlns="http://maven.apache.org/SETTINGS/1.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/SETTINGS/1.0.0
http://maven.apache.org/xsd/settings-1.0.0.xsd">
<localRepository/>
<interactiveMode/>
<usePluginRegistry/>
<offline/>
<pluginGroups/>
<servers>
<server>
<id>azure-auth</id>
<configuration>
<client>ur key</client>
<tenant>ur tenant</tenant>
<key>YOUR PASSWORD</key>
<environment>AZURE</environment>
</configuration>
</server>
</servers>
<proxies/>
<profiles>
<profile>
<id>hwx</id>
<repositories>
<repository>
<id>hwx</id>
<name>hwx</name>
<url>http://nexus-private.hortonworks.com/nexus/content/groups/public/</url>
</repository>
</repositories>
</profile>
</profiles>
<mirrors>
<mirror>
<id>public</id>
<mirrorOf>*</mirrorOf>
<url>http://nexus-private.hortonworks.com/nexus/content/groups/public/</url>
</mirror>
</mirrors>
<activeProfiles/>
</settings>
2) Add your Azure service principal settings from the previous section of this tutorial to the collection in the settings.xml file as shown below:
<servers>
<server>
<id>azure-auth</id>
<configuration>
<client>aaaaaaaa-aaaa-aaaa-aaaa-aaaaaaaaaaaa</client>
<tenant>tttttttt-tttt-tttt-tttt-tttttttttttt</tenant>
<key>pppppppp</key>
<environment>AZURE</environment>
</configuration>
</server>
</servers>
3) Save and close the settings.xml file.
Build and deploy your app to Azure
1) run following command
- mvn azure-webapp:deploy
When your web app has been deployed, visit the Azure portal to manage
it. It will be listed in App Services.
Click on the application. From there, the publicly-facing URL for
your web app will be listed in the Overview section
Determining the URL for your web app You can click on this link to
visit the Spring Boot application and interact with it.
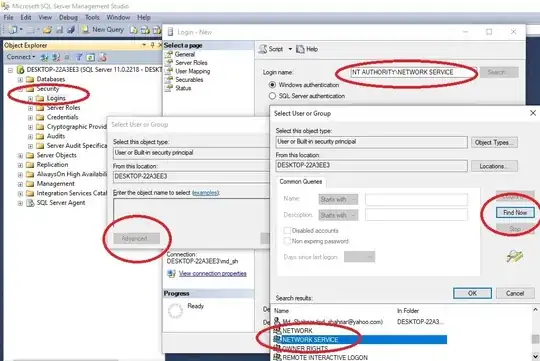
Azure maven plugin doc
Note : The website name has to be globally unique and its generated
using app name , make sure name should be unique.