I need to ask this question and I know this might get mark as duplicate since so many people already ask the similar questions but I have read through them and find no answers or similarities to the problem that I'm facing.
Trying to get property of non-object
Okay, right now, I have a table that will show the list of a user for admin to add, edit or delete the user. My problem right now is, the table can only display the users list and when I add the edit and delete part, the notice pops up.
This is my code before alteration of edit and delete;
<?php
$db_host = 'localhost'; // Server Name
$db_user = 'root'; // Username
$db_pass = ''; // Password
$db_name = 'register'; // Database Name
$conn = mysqli_connect($db_host, $db_user, $db_pass, $db_name);
if (!$conn) {
die ('Failed to connect to MySQL: ' . mysqli_connect_error());
}
$sql = 'SELECT *
FROM users';
$query = mysqli_query($conn, $sql);
if (!$query) {
die ('SQL Error: ' . mysqli_error($conn));
}
?>
<table class="data-table">
<caption class="title">List of Users </caption>
<thead>
<tr>
<th>USERNAME</th>
<th>PASSWORD</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody>
<?php
$no = 1;
$total = 0;
while ($row = mysqli_fetch_array($query))
{
echo '<tr>
<td>'.$row['username'].'</td>
<td>'.$row['password'].'</td>
</tr>';
}?>
</tbody>
</table>
and here is my code after the alteration
<?php
$db_host = 'localhost'; // Server Name
$db_user = 'root'; // Username
$db_pass = ''; // Password
$db_name = 'register'; // Database Name
$conn = mysqli_connect($db_host, $db_user, $db_pass, $db_name);
if (!$conn) {
die ('Failed to connect to MySQL: ' . mysqli_connect_error());
}
$sql = 'SELECT *
FROM users';
$query = mysqli_query($conn, $sql);
if (!$query) {
die ('SQL Error: ' . mysqli_error($conn));
}
?>
<table class="data-table">
<caption class="title">List of Users </caption>
<thead>
<tr>
<th>USERNAME</th>
<th>PASSWORD</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody>
<?php
$no = 1;
$total = 0;
while ($row = mysqli_fetch_array($query))
{
echo "<tr>";
echo "<td>" . $row->username . "</td>";
echo "<td>" . $row->password . "</td>";
echo "<td><a href='records.php?id=" . $row->id . "'>Edit</a></td>";
echo "<td><a href='delete.php?id=" . $row->id . "'>Delete</a></td>";
echo "</tr>";
}?>
</tbody>
</table>
</div>
</body>
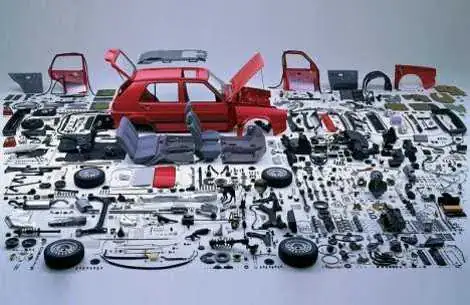
Where did I do wrong and how can I solve my problem? Every suggestion and hints are greatly appreciated and please don't mark my question since I believe that my question is totally different from the others.