You have to split this value based on spaces
and return only fields that starts with a colon :
, i provided 2 solutions to achieve this based on the result type you need (Table or Single Value)
Table-Valued Function
You can create a TV function to split this column into a table:
CREATE FUNCTION [dbo].[GETVALUES]
(
@DelimitedString varchar(8000)
)
RETURNS @tblArray TABLE
(
ElementID int IDENTITY(1,1), -- Array index
Element varchar(1000) -- Array element contents
)
AS
BEGIN
-- Local Variable Declarations
-- ---------------------------
DECLARE @Index smallint,
@Start smallint,
@DelSize smallint
SET @DelSize = 1
-- Loop through source string and add elements to destination table array
-- ----------------------------------------------------------------------
WHILE LEN(@DelimitedString) > 0
BEGIN
SET @Index = CHARINDEX(' ', @DelimitedString)
IF @Index = 0
BEGIN
IF ((LTRIM(RTRIM(@DelimitedString))) LIKE ':%')
INSERT INTO
@tblArray
(Element)
VALUES
(LTRIM(RTRIM(@DelimitedString)))
BREAK
END
ELSE
BEGIN
IF (LTRIM(RTRIM(SUBSTRING(@DelimitedString, 1,@Index - 1)))) LIKE ':%'
INSERT INTO
@tblArray
(Element)
VALUES
(LTRIM(RTRIM(SUBSTRING(@DelimitedString, 1,@Index - 1))))
SET @Start = @Index + @DelSize
SET @DelimitedString = SUBSTRING(@DelimitedString, @Start , LEN(@DelimitedString) - @Start + 1)
END
END
RETURN
END
And you can use it like the following:
DECLARE @SQLStr varchar(100)
SELECT @SQLStr = 'abc = :abc and this = :that and xyz = :asd'
SELECT
*
FROM
dbo.GETVALUES(@SQLStr)
Result:
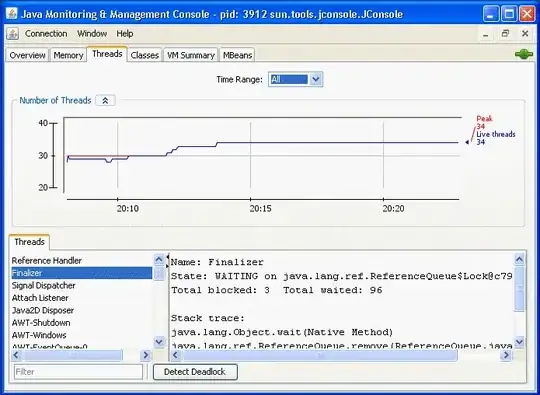
Scalar-Valued Function
If you need to return a value (not table) so you can use this function which will return on all values separated by (line feed + carridge return CHAR(13) + CHAR(10)
)
CREATE FUNCTION dbo.GetValues2
(
@DelimitedString varchar(8000)
)
RETURNS varchar(8000)
AS
BEGIN
DECLARE @Index smallint,
@Start smallint,
@DelSize smallint,
@Result varchar(8000)
SET @DelSize = 1
SET @Result = ''
WHILE LEN(@DelimitedString) > 0
BEGIN
SET @Index = CHARINDEX(' ', @DelimitedString)
IF @Index = 0
BEGIN
if (LTRIM(RTRIM(@DelimitedString))) LIKE ':%'
SET @Result = @Result + char(13) + char(10) + (LTRIM(RTRIM(@DelimitedString)))
BREAK
END
ELSE
BEGIN
IF (LTRIM(RTRIM(SUBSTRING(@DelimitedString, 1,@Index - 1)))) LIKE ':%'
SET @Result = @Result + char(13) + char(10) + (LTRIM(RTRIM(SUBSTRING(@DelimitedString, 1,@Index - 1))))
SET @Start = @Index + @DelSize
SET @DelimitedString = SUBSTRING(@DelimitedString, @Start , LEN(@DelimitedString) - @Start + 1)
END
END
return @Result
END
GO
you can use it as the following
DECLARE @SQLStr varchar(100)
SELECT @SQLStr = 'abc = :abc and this = :that and xyz = :asd'
SELECT dbo.GetValues2(@SQLStr)
Result
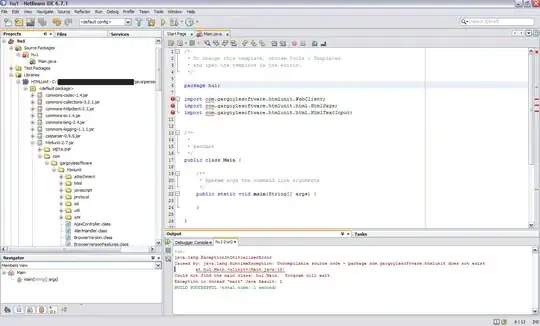
in the table result line feed are not visible, just copy the data to an editor and it will appears as shown in the image
References