I think to approach this problem it may be necessary to see what they are using to parse the input of the user. If they are using DateTime.Parse then it will throw an exception when the string being parsed is ambiguous.
Of course, a programmer could always create their own way of parsing the input in the field. Though, typically a programmer isn't that enthusiastic about dealing with the ambiguous cases when parsing information. So let's assume they are working with a DateTime.Parse method for simplicity sake.
I created the following program to allow you to see when the parser sees something as ambiguous. The output of the program is shown in this picture:
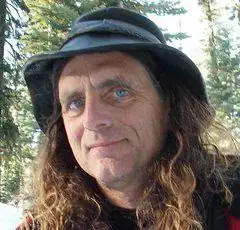
The code demonstrating DateTime.Parse:
static void Main(string[] args)
{
string input = "";
while(input != "exit" || input != "Exit")
{
Console.Write("Input: ");
input = Console.ReadLine();
string inputDate = input;
//try to parse it
try
{
DateTime date = DateTime.Parse(inputDate);
Console.WriteLine(date+"\n");
}
catch
{
//Exceptions. String not valid because ambiguity
Console.WriteLine("Ambiguous.\n");
//In here can also perform other logic, of course
}
}
}
In order to convert the DateTime back to a string you can do something similar to:
try
{
DateTime date = DateTime.Parse(inputDate);
Console.WriteLine(date+"\n");
string month = date.Month.ToString();
string day = date.Day.ToString();
string year = date.Year.ToString();
string resultingString = month + " " + day + " " + year ;
Console.WriteLine(resultingString);
}
catch(Exception e)
{
//Exceptions. String not valid because ambiguity
Console.WriteLine("Ambiguous");
}
You can even make your own parser with this information in this fashion so you can acheive a result for a date entered that is 3 characters long:
static void Main(string[] args)
{
string input = "";
while(input != "exit" || input != "Exit")
{
Console.Write("Input: ");
input = Console.ReadLine();
string inputDate = input;
try
{
DateTime date = DateTime.Parse(inputDate);
Console.WriteLine(date+"\n");
string month = date.Month.ToString();
string day = date.Day.ToString();
string year = date.Year.ToString();
string resultingString = month + " " + day + " " + year;
//string resultingString = month + "/" + day + "/" + year;
Console.WriteLine(resultingString);
}
catch(Exception e)
{
//Exceptions. String not valid because ambiguity
try
{
Console.WriteLine( myParser(inputDate) );
}
catch
{
Console.WriteLine("Ambiguous");
}
//Console.WriteLine("Ambiguous.\n");
//In here can also perform other logic, of course
}
}
}
static string myParser(string input)
{
string month,day,year,date;
switch (input.Length)
{
//In the case that it's 1 character in length
case 1:
return "Ambiguous.";
case 2:
return "Ambiguous.";
//did user mean #/#/200#? hopefully not #/#/199#...
case 3:
month = input.Substring(0, 1);
day = input.Substring(1, 1);
year = input.Substring(2, 1);
date = month + " " + day + " " + year;
return date;
//did user mean # # 20##
//or # ## 200#
//or ## # 200#
case 4:
return "Ambiguous";
//user probably means ## # ##
case 5:
return "Ambiguous";
case 6:
return "Ambiguous";
default:
return "Ambiguous";
}
}
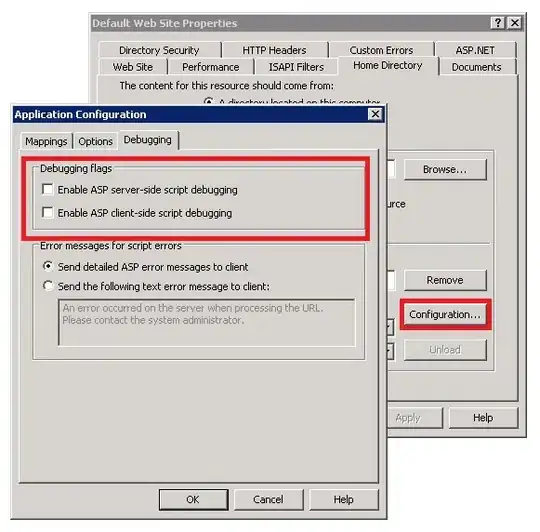
Similarly, if you want to get the date back to a slash (/
) seperated format in the form of a string without the minutes and hours and such..
case 3:
month = input.Substring(0, 1);
day = input.Substring(1, 1);
year = input.Substring(2, 1);
date = month + " " + day + " " + year;
DateTime dateTimeObj = DateTime.Parse(date);
month = dateTimeObj.Month.ToString();
day = dateTimeObj.Day.ToString();
year = dateTimeObj.Year.ToString();
string resultingString = month + "/" + day + "/" + year;
return resultingString;
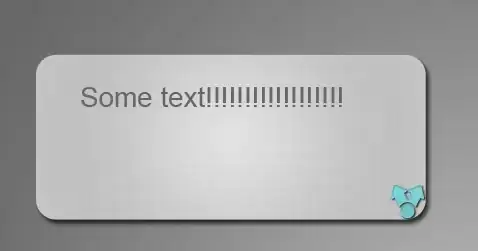