It is not possible to insert plots into a matplotlib table. However subplot grids allow to create a table-like behaviour.
import matplotlib.pyplot as plt
import numpy as np
data = np.random.rand(100,4)
col1 = ["WAR", "ERA", "IP", "WHIP", "Final\nScore"]
col2 = [0.23,1.60,0.28,0.02,0.38]
col2colors = ["red", "g", "r", "r", "r"]
finalsc = "D+"
fig, axes = plt.subplots(ncols=3, nrows=5, figsize=(4,2.6),
gridspec_kw={"width_ratios":[1,0.5,2]})
fig.subplots_adjust(0.05,0.05,0.95,0.95, wspace=0.05, hspace=0)
for ax in axes.flatten():
ax.tick_params(labelbottom=0, labelleft=0, bottom=0, top=0, left=0, right=0)
ax.ticklabel_format(useOffset=False, style="plain")
for _,s in ax.spines.items():
s.set_visible(False)
border = fig.add_subplot(111)
border.tick_params(labelbottom=0, labelleft=0, bottom=0, top=0, left=0, right=0)
border.set_facecolor("None")
text_kw = dict(ha="center", va="bottom", size=13)
for i,ax in enumerate(axes[:,0]):
ax.text(0.5, 0.05, col1[i], transform=ax.transAxes, **text_kw)
for i,ax in enumerate(axes[:,1]):
ax.text(0.5, 0.05, "{:.2f}".format(col2[i]),transform=ax.transAxes, **text_kw)
ax.set_facecolor(col2colors[i])
ax.patch.set_color(col2colors[i])
axes[-1,-1].text(0.5, 0.05, finalsc,transform=axes[-1,-1].transAxes, **text_kw)
for i,ax in enumerate(axes[:-1,2]):
ax.plot(data[:,i], color="green", linewidth=1)
plt.show()

To put several such plots into a figure you would approach this a bit differently and create a gridspec with several subgrids.
import matplotlib.pyplot as plt
from matplotlib import gridspec
import numpy as np
def summaryplot2subplot(fig, gs, data, col1, col2, finalsc):
col2colors = ["g" if col2[i] > 1 else "r" for i in range(len(col2)) ]
sgs = gridspec.GridSpecFromSubplotSpec(5,3, subplot_spec=gs, wspace=0.05, hspace=0,
width_ratios=[0.9,0.7,2])
axes = []
for n in range(5):
for m in range(3):
axes.append(fig.add_subplot(sgs[n,m]))
axes = np.array(axes).reshape(5,3)
for ax in axes.flatten():
ax.tick_params(labelbottom=0, labelleft=0, bottom=0, top=0, left=0, right=0)
ax.ticklabel_format(useOffset=False, style="plain")
for _,s in ax.spines.items():
s.set_visible(False)
border = fig.add_subplot(gs)
border.tick_params(labelbottom=0, labelleft=0, bottom=0, top=0, left=0, right=0)
border.set_facecolor("None")
text_kw = dict(ha="center", va="bottom", size=11)
for i,ax in enumerate(axes[:,0]):
ax.text(0.5, 0.05, col1[i], transform=ax.transAxes, **text_kw)
for i,ax in enumerate(axes[:,1]):
ax.text(0.5, 0.05, "{:.2f}".format(col2[i]),transform=ax.transAxes, **text_kw)
ax.set_facecolor(col2colors[i])
ax.patch.set_color(col2colors[i])
axes[-1,-1].text(0.5, 0.05, finalsc,transform=axes[-1,-1].transAxes, **text_kw)
for i,ax in enumerate(axes[:-1,2]):
ax.plot(data[:,i], color=col2colors[i], linewidth=1)
fig = plt.figure(figsize=(8,6))
gs = gridspec.GridSpec(2,2)
col1 = ["WAR", "ERA", "IP", "WHIP", "Final\nScore"]
finalsc = "D+"
for i in range(4):
data = np.random.rand(100,4)
col2 = np.random.rand(5)*2
summaryplot2subplot(fig, gs[i], data, col1, col2, finalsc)
plt.show()
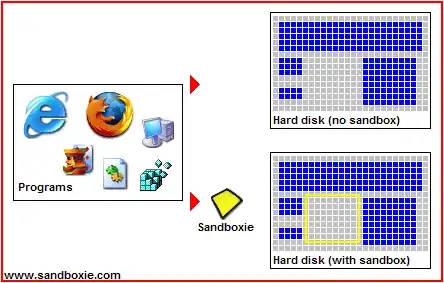