I found out the solution, as lack of time I tried for Apple Map, but you try for google map also.
Steps
- Get the location where you wanted to show annotation.
- Add this point annotation on Map.
- Create a
UILabel
(says, lbl
) with text as you wanted.
- Add this text on a view (says,
viewAn
).
- Now capture the
viewAn
and make it image.
- Use this image for location marker.
Below is the code work for Apple Map and out of simulator is added below it and it is working properly. Follow the above steps and definatly it will work for google map also.
Code Work
import UIKit
import MapKit
class MapVC: UIViewController, MKMapViewDelegate {
@IBOutlet weak var mapView: MKMapView! // Apple mapview Outlet
var location: CLLocationCoordinate2D = CLLocationCoordinate2D(latitude: 28.5961279, longitude: 77.1587375)
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
let anno = MKPointAnnotation();
anno.coordinate = location;
mapView.addAnnotation(anno);
}
// To capture view
func captureScreen(_ viewcapture : UIView) -> UIImage {
UIGraphicsBeginImageContextWithOptions(viewcapture.frame.size, viewcapture.isOpaque, 0.0)
viewcapture.layer.render(in: UIGraphicsGetCurrentContext()!)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return image!;
}
func mapView(_ mapView: MKMapView, viewFor annotation: MKAnnotation) -> MKAnnotationView? {
// Don't want to show a custom image if the annotation is the user's location.
guard !(annotation is MKUserLocation) else {
return nil
}
// Better to make this class property
let annotationIdentifier = "AnnotationIdentifier"
var annotationView: MKAnnotationView?
if let dequeuedAnnotationView = mapView.dequeueReusableAnnotationView(withIdentifier: annotationIdentifier) {
annotationView = dequeuedAnnotationView
annotationView?.annotation = annotation
}
else {
annotationView = MKAnnotationView(annotation: annotation, reuseIdentifier: annotationIdentifier)
annotationView?.rightCalloutAccessoryView = UIButton(type: .detailDisclosure)
}
if let annotationView = annotationView {
// Configure your annotation view here
// view for annotation
let viewAn = UIView()
viewAn.frame = CGRect(x: 0, y: 0, width: 80, height: 18)
// label as required
let lbl = UILabel()
lbl.text = "ABC 123"
lbl.textColor = UIColor.black
lbl.backgroundColor = UIColor.cyan
// add label to viewAn
lbl.frame = viewAn.bounds
viewAn.addSubview(lbl)
// capture viewAn
let img = self.captureScreen(viewAn)
annotationView.canShowCallout = true
// set marker
annotationView.image = img
}
return annotationView
}
}
OutPut :
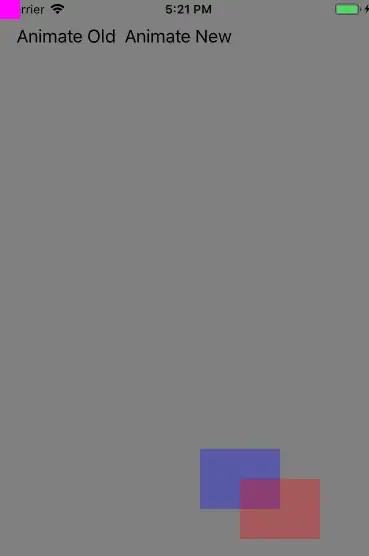
Edit : image trasparency
use this below func
func changeWhiteColorTransparent(_ image: UIImage) -> UIImage {
let rawImageRef = image.cgImage as! CGImage
let colorMasking : [CGFloat] = [222, 255, 222, 255, 222, 255]
UIGraphicsBeginImageContext(image.size)
let maskedImageRef: CGImage = rawImageRef.copy(maskingColorComponents: colorMasking)!
do {
//if in iphone
UIGraphicsGetCurrentContext()?.translateBy(x: 0.0, y: image.size.height)
UIGraphicsGetCurrentContext()?.scaleBy(x: 1.0, y: -1.0)
}
UIGraphicsGetCurrentContext()?.draw(maskedImageRef, in: CGRect(x: 0, y: 0, width: image.size.width, height: image.size.height))
let result = UIGraphicsGetImageFromCurrentImageContext() as! UIImage
UIGraphicsEndImageContext()
return result ?? UIImage()
}
Func callie
Replace code of upper annotationview with below
let viewAn = UIView()
viewAn.frame = CGRect(x: 0, y: 0, width: 80, height: 18)
let lbl = UILabel()
lbl.text = "ABC 123"
lbl.textColor = UIColor.black
lbl.backgroundColor = UIColor.clear
viewAn.backgroundColor = UIColor.white
lbl.frame = viewAn.bounds
viewAn.addSubview(lbl)
let img = self.captureScreen(viewAn)
let aImgNew = self.changeWhiteColorTransparent(img)
annotationView.backgroundColor = UIColor.clear
annotationView.canShowCallout = true
annotationView.image = aImgNew
Output:
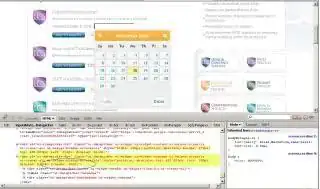