EDIT
I deleted everything. Use this two new methods:
def FindMax( listOfY , indexes, numOfMax):
'This method finds the maximum values in the list of peaks'
listMax = []
xList = []
reconstructedList = []
for c in range(0,numOfMax):
listMax.append(max(listOfY))
index = listOfY.index(max(listOfY))
xList.append(indexes[index])
listOfY.pop(index)
return listMax, xList
def FindPeaks(listY):
'This method finds the peaks from the list with the Y values'
peaks = []
indexes = []
count = 0
m2 = 0 #Old slope: starts with 0
for value in range(1,len(listY)):
m1 = listY[value] - listY[value-1] #New slope
if( m2 > 0 and m1 < 0 ):
peaks.append(listY[value-1])
indexes.append( value-1 )
m2 = m1 #Old slope is assigned
return peaks, indexes
#Test
list = [1,3,55,5,76,26,77,88,4,96,1,5,2,7,3,100,100,100,76,25]
peaksList = FindPeaks(list) #List: (['peaks'],['indexes'])
print ("List: " , list)
print ("Peaks list: ",peaksList[0])
print ("Peaks indexes: ",peaksList[1])
maxList = FindMax(peaksList[0],peaksList[1],3)
print ("Max: ", maxList[0])
print ("Max indexes: ", maxList[1])
peaksList = FindPeaks(list)
print ("Peaks: ",peaksList)
FindPeaks() method will use your list with the Y values as argument and won't modify it, it also returns a 2D list, where the first index of that list is the peaks list, and second list their index in "list". After that, the peaksList[0], peaksList1, and the number of max peaks you want are passed as argument to the method FindMax(), returning a 2D list. This new list has in the index '0' a list containing the maximum peaks, in descending order, at the index '1' of peaksList are the indexes where you find them at List 'list', which is your inicial list.
Below the comment #Test you can find all the tests I ran. As you can see this method can't detect flat peaks, but you could pass as an argument to the method FindMax() the whole list, with all the arguments described above as well and you will get all the values in the flat peak, which will look as a sequence of the same value ( [...',100,100,100'...] ). But the method FindPeaks() will be usefull when finding all other peaks (not flat peaks as I described), so in the case you have a flat peak this method won't return it.
Output command-line window
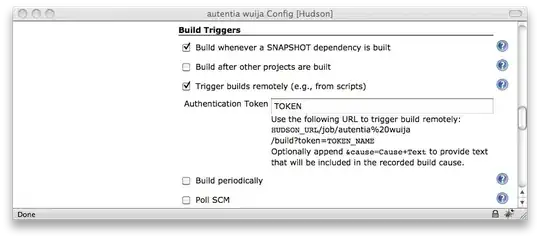
I hope you find this useful for you.