Solution:
As Heinzi stated in a comment, you should just use the RunWorkerCompleted event.
Here is an good example on "how to use the backgroundworker runworkercompleted event" You shall never "wait" for a task/thread to finish, but you could "wait" for waithandles or let the thread do other stuff in the meantime
In the Class where you hold an instance of the
Backgroundworker
you can simply attach to the completed event and your fine.
Delete that loop with the Thread.Sleep() in it
.
private void WorkerExample() {
BackgroundWorker bw = new BackgroundWorker();
bw.RunWorkerCompleted += OnWorkerCompleted; //listen for that event by attaching a handler
bw.RunWorkerAsync();
}
//This is the EventHandler
private void OnWorkerCompleted(object sender, RunWorkerCompletedEventArgs runWorkerCompletedEventArgs) {
//This method is called, when the worker finished his job
}
Further Information:
There are also some more useful events on the BackgroundWorker.
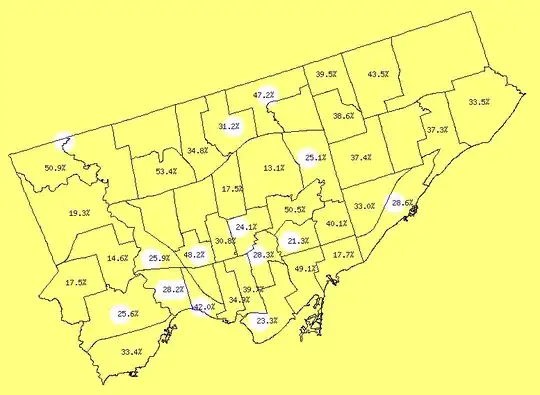
MSDN provides a complete example how to use the BackgroundWorker. You should have a look at that.