Firstly, you can employ Canny edge detection method to find all edges.
Secondly, it's better to use a high value of the sensitivity to find all circular-like shapes and then restrict your conditions to determine the right number of circles. For instance, you can utilize the distance of the circle centers (or correlations of circles). Here's my code to determine the number coins in the given image.
clc;clear all;close all;
%% Preporocessing
input = imread('img.jpg');
input_edges = edge(input,'Canny');
figure;imshow(input_edges);
input_edges=medfilt2(input_edges,[2 2]);% with this filter size, the edges can be strengthened!
figure;imshow(input_edges);
input_edges=bwareaopen(input_edges,130);%remove small edges
%% Applying circular Hough transform
[centres, radii, metric] = imfindcircles(input_edges, [35, 90],...
'ObjectPolarity','bright','Sensitivity',0.9,'EdgeThreshold',0.1);
figure;imshow(input_edges);
[l,c] = size(radii);
hold on;
plot(centres(:,1), centres(:,2), 'r*');
viscircles(centres, radii, 'EdgeColor', 'b');
%% Distinguishing circlar shadow
x_centres=centres(:,1);
y_centres=centres(:,2);
num=size(centres,1);
centre_dists = sqrt( bsxfun(@minus,centres(:,1),centres(:,1)').^2 + ...
bsxfun(@minus,centres(:,2),centres(:,2)').^2 );
% [x_idx,y_idx] =find(centre_dists<50 & centre_dists>0);
% sort(centre_dists(centre_dists<50 & centre_dists>0));
coin_nm=size(centres,1)-0.5*numel(find(centre_dists<50 & centre_dists>0));
The output is obtained as below:
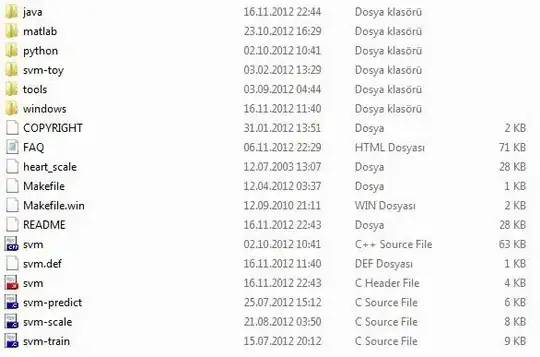