Try this and see:
@IBOutlet weak var loginButton: UIButton!
func testGradientButton() -> Void {
let gradientColor = CAGradientLayer()
gradientColor.frame = loginButton.frame
gradientColor.colors = [UIColor.blue.cgColor,UIColor.red.withAlphaComponent(1).cgColor]
self.loginButton.layer.insertSublayer(gradientColor, at: 0)
}
Here is result:
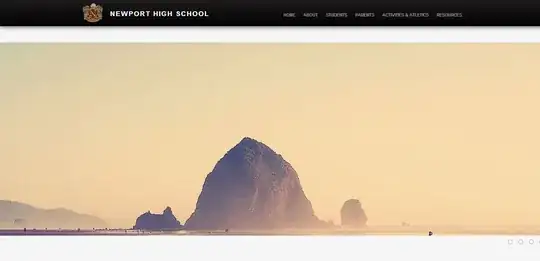
Also note: Values for RGB colors in your code, are much higher than its normal value. See your console log, it might printed following error:
[Graphics] UIColor created with component values far outside the expected range. Set a breakpoint on UIColorBreakForOutOfRangeColorComponents to debug. This message will only be logged once.
Divide all values with 255.0 and use insertSubLayer
function.
let color1 = UIColor(red: 183.0/255.0, green: 46.0/255.0, blue: 79.0/255.0, alpha: 1)
let color2 = UIColor(red: 232.0/255.0, green: 79.0/255.0, blue: 80.0/255.0, alpha: 1)
let color3 = UIColor(red: 145.0/255.0, green: 21.0/255.0, blue: 79.0/255.0, alpha: 1)
Here is result with your color code values:
func testGradientButton() -> Void {
let gradientColor = CAGradientLayer()
gradientColor.frame = loginButton.frame
let color1 = UIColor(red: 183.0/255.0, green: 46.0/255.0, blue: 79.0/255.0, alpha: 1)
let color2 = UIColor(red: 232.0/255.0, green: 79.0/255.0, blue: 80.0/255.0, alpha: 1)
let color3 = UIColor(red: 145.0/255.0, green: 21.0/255.0, blue: 79.0/255.0, alpha: 1)
gradientColor.colors = [color1.cgColor,color2.cgColor,color3.cgColor]
self.loginButton.layer.insertSublayer(gradientColor, at: 0)
}
