There is a bug in Firebase SDK 11.8.0 on Android 8.0 (API 26), which causes the display of a white version of the app's launcher icon in the status bar instead of the notification icon.
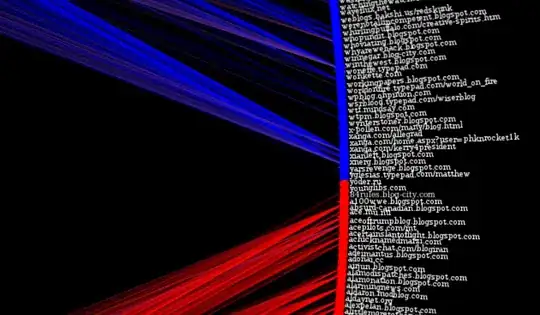
Some people have fixed it by overriding the Application class's getResources() method.
Another way that worked for me was to use an HTTP POST request to send the notification as a data message:
https://fcm.googleapis.com/fcm/send
Content-Type:application/json
Authorization:key=AIzaSyZ-1u...0GBYzPu7Udno5aA
{
"to": "/topics/test",
"data": {
"title": "Update",
"body": "New feature available"
}
}
And then subclass FirebaseMessagingService to display the notification programmatically:
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
Intent i = new Intent(context, HomeActivity.class);
PendingIntent pi = PendingIntent.getActivity(this, 0, i,
PendingIntent.FLAG_ONE_SHOT);
NotificationCompat.Builder builder =
new NotificationCompat.Builder(this, GENERAL_CHANNEL_ID)
.setSmallIcon(R.drawable.ic_stat_chameleon)
.setContentTitle(remoteMessage.getData().get("title"))
.setContentText(remoteMessage.getData().get("body"))
.setContentIntent(pi);
NotificationManager manager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
manager.notify(0, builder.build());
}
}
In the end, I got this, even on Android 8.0:
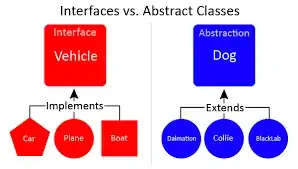