It is rather straightforward to import features from later versions of Three.js even if the Viewer version is behind. I used the threejs-full-es6 package which allows to import only what's needed from the version of Three.js, just so you don't bloat your app with two versions of the lib and also prevents namespace collision. It is using r89 at the moment, another good news!
Here is a simple ES6 extension I created that wraps the TextGeometry creation:
import { Font, TextGeometry } from 'threejs-full-es6'
import FontJson from './helvetiker_bold.typeface.json'
export default class TestExtension
extends Autodesk.Viewing.Extension {
constructor (viewer, options) {
super()
this.viewer = viewer
}
load () {
return true
}
unload () {
return true
}
/////////////////////////////////////////////////////////
// Adds a color material to the viewer
//
/////////////////////////////////////////////////////////
createColorMaterial (color) {
const material = new THREE.MeshPhongMaterial({
specular: new THREE.Color(color),
side: THREE.DoubleSide,
reflectivity: 0.0,
color
})
const materials = this.viewer.impl.getMaterials()
materials.addMaterial(
color.toString(),
material,
true)
return material
}
/////////////////////////////////////////////////////////
// Wraps TextGeometry object and adds a new mesh to
// the scene
/////////////////////////////////////////////////////////
createText (params) {
const geometry = new TextGeometry(params.text,
Object.assign({}, {
font: new Font(FontJson),
params
}))
const material = this.createColorMaterial(
params.color)
const text = new THREE.Mesh(
geometry , material)
text.position.set(
params.position.x,
params.position.y,
params.position.z)
this.viewer.impl.scene.add(text)
this.viewer.impl.sceneUpdated(true)
}
}
Autodesk.Viewing.theExtensionManager.registerExtension(
'Viewing.Extension.Test', TestExtension)
To use it, simply load the extension and invoke createText
method:
import './Viewing.Extension.Test'
// ...
viewer.loadExtension('Viewing.Extension.Test').then((extension) => {
extension.createText({
position: {x: -150, y: 50, z: 0},
bevelEnabled: true,
curveSegments: 24,
bevelThickness: 1,
color: 0xFFA500,
text: 'Forge!',
bevelSize: 1,
height: 1,
size: 1
})
})
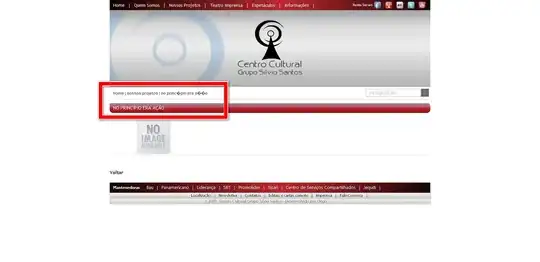
Sweet! ;)