Since I cant comment because I dont have 50 reputation, I had to write it here, its hard to tell from the code you posted, to give you an answer I need more detail on what getAddressIdPostCodeList()
does, since you said the data in the DB has no ceros the error must be in the method getAddressIdPostCodeList()
and/or in how you are handling the resultset
of the Query
EDIT: Solution using Oracle JDBC Driver
Since I dont know how to use Mybatis
, heres a solution using JDBC
.
to connect using JDBC to your Oracle
DB here's a simple tutorial:
1. First you need to download de JDBC
driver from Oracle depending your DB version (11g,12c,10g), the driver Its called ojdbcX.jar
where X is a number of the version of the driver
2. After you have downloaded the driver you need to add the .jar
to your project, if you are using Netbeans IDE you can add it like this:
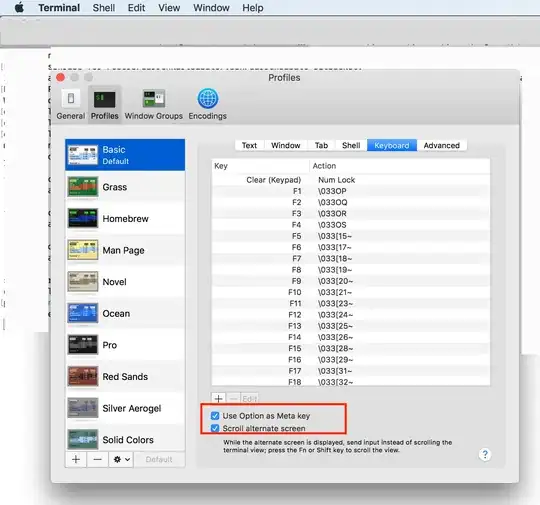
if you are using Eclipse you can use the following Link to see how to add the .JAR
file: How to import a jar in Eclipse
3. After adding the .JAR
its pretty simple, you just need to connect to the DB using your credentials, here is an example on how to do it:
Connection connection = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connection = DriverManager.getConnection(
"jdbc:oracle:thin:@localhost:1521:xe", "system", "password"); } catch (Exception ex) {
ex.printStackTrace();
}
for more deatiled information on how to connect, you can check the oracle.jdbc
Class OracleDriver Documentation
4. After the connection has been made its pretty simple, Heres a short code example to get the result you want, you need to modify it with your connection details and as you see fit because im making a couple of assumptions, this code is just an example:
Main.Java
public class Main {
public static void main(String[] argv) {
List<AddressID> addresses;
SQLConnect conex= new SQLConnect();
String caseid="the id you want";
addresses=conex.getAddressIdPostCodeList(caseid);
}
AddressID.java
public class AddressID {
private String addressId;
private String postcode;
}
SQLConnect.Java
public class SQLConnect {
public static Connection connection;
public SQLConnect (){
createConnection();
}
public void CreateConnection(){
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connection = DriverManager.getConnection(
"jdbc:oracle:thin:@localhost:1521:xe", "system", "password")
} catch (Exception ex) {
ex.printStackTrace();
}
}
public void closeConnection(){
if(connection!=null){
try {
connection.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
public ResultSet ExecuteQuery(String queryTXT) throws SQLException{
Statement query = connection.createStatement();
ResultSet table=query.executeQuery(queryTXT);
return table;
}
public List<AddressID> getAddressIdPostCodeList(String caseid) throws SQLException{
List<AddressID> addresses = new ArrayList <> ();
ResultSet table=ExecuteQuery("SELECT address_id,postcode FROM table1 WHERE custom_field_1 ='"+caseid+"';");
while (table.next()) {
AddressID aux;
aux.addressId=table.getString(1);
aux.postcode=table.getString(2);
addresses.add(aux);
}
return addresses;
}
}