You can use progressbar
like this:
import time
from progressbar import ProgressBar
pbar = ProgressBar()
def job():
for i in pbar(xrange(5)):
print(i)
job()
Output looks like this:
0 0% | |
120% |############## |
240% |############################# |
360% |########################################### |
480% |########################################################## |
100% |#########################################################################
I like tqdm a lot more and it works the same way.
from tqdm import tqdm
for i in tqdm(range(10000)):
pass
Image
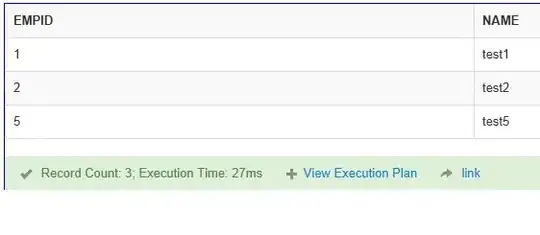