Here's a way to use SciPy's radial basis function interpolator. Omit the %matplotlib inline
magic if you're not in a notebook.
import numpy as np
from scipy.interpolate import Rbf
%matplotlib inline
import matplotlib.pyplot as plt
data = {(1,3):22, (1,3.5):23, (1,4.5):25, (1.5,3.3):19, (4,4):100}
# Extract the data.
x = np.array([k[0] for k in data.keys()])
y = np.array([k[1] for k in data.keys()])
z = np.array([v for v in data.values()])
# Make the grid.
minx, maxx = np.amin(x), np.amax(x)
miny, maxy = np.amin(y), np.amax(y)
extent = (minx, maxx, miny, maxy)
grid_x, grid_y = np.mgrid[minx:maxx:0.01, miny:maxy:0.01]
# Make an n-dimensional interpolator.
rbfi = Rbf(x, y, z)
# Predict on the regular grid.
z_ = rbfi(grid_x, grid_y)
# Look at it!
plt.imshow(z_, origin="lower", extent=extent)
plt.scatter(x, y, s=2, c='w')
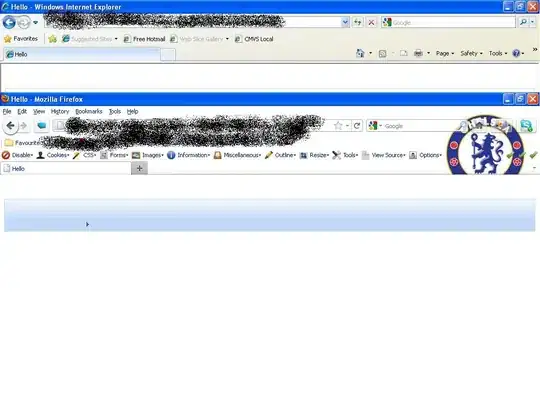