There are a number of ways to do this, I prefer using the CALayer
of a UIView
and adding a CAShapeLayer
that draws the "progress bar".
UIView ProgressBar Example:
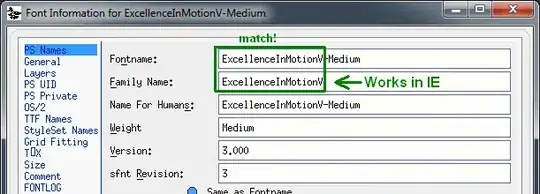
public class ProgressView : UIView
{
CAShapeLayer progressLayer;
UILabel label;
public ProgressView() { Setup(); }
public ProgressView(Foundation.NSCoder coder) : base(coder) { Setup(); }
public ProgressView(Foundation.NSObjectFlag t) : base(t) { Setup(); }
public ProgressView(IntPtr handle) : base(handle) { }
public ProgressView(CGRect frame) : base(frame) { Setup(); }
void Setup()
{
BackgroundColor = UIColor.Clear;
Layer.CornerRadius = 25;
Layer.BorderWidth = 10;
Layer.BorderColor = UIColor.Blue.CGColor;
Layer.BackgroundColor = UIColor.Cyan.CGColor;
progressLayer = new CAShapeLayer()
{
FillColor = UIColor.Red.CGColor,
Frame = Bounds
};
Layer.AddSublayer(progressLayer);
label = new UILabel(Bounds)
{
TextAlignment = UITextAlignment.Center,
TextColor = UIColor.White,
BackgroundColor = UIColor.Clear,
Font = UIFont.FromName("ChalkboardSE-Bold", 20)
};
InsertSubview(label, 100);
}
double complete;
public double Complete
{
get { return complete; }
set { complete = value; label.Text = $"{value * 100} %"; SetNeedsDisplay(); }
}
public override void Draw(CGRect rect)
{
base.Draw(rect);
var progressWidth = (rect.Width - (Layer.BorderWidth * 2)) * complete;
var progressRect = new CGRect(rect.X + Layer.BorderWidth, rect.Y + Layer.BorderWidth, progressWidth, (rect.Height - Layer.BorderWidth * 2));
progressLayer.Path = UIBezierPath.FromRoundedRect(progressRect, 25).CGPath;
}
}
Usage Example:
var progressView = new ProgressView(new CGRect(50, 50, 300, 50));
Add(progressView);
DispatchQueue.MainQueue.DispatchAsync(async () =>
{
while (progressView.Complete <= 1)
{
InvokeOnMainThread(() => progressView.Complete += 0.05);
await Task.Delay(1000);
}
});