If you want to have toggleable lines of code, you can do something like this, example being a debug/trace implementation.
@Echo Off
::Set trace bit
set trace=0
::Optional: Prompt to enable
set /p trace=Trace is disabled. Enter 1 to enable:
::Implement trace bit
if %trace% EQU 1 (
set trace=
) else (
set trace=@REM
)
::Example Script (Lines that begin with %trace% are dependent on trace bit)
%trace% @Echo On
set x=5
%trace% @Echo Trace1: x=%x%
set /a y=5*%x%
%trace% @Echo Trace2: y=%y%
Echo On
set /a y=5*%y%
%trace% @Echo Trace3: y=%y%
pause
Explanation
This technique works by setting a parameter (%trace% in this example) to be a non-printing comment @REM
if trace is off (= 0). This in effect masks your entire line of code by making it appear as a comment. If trace is enabled (= 1), then the %trace% variable is empty and the entire line of code executes as normal.
Line Masking Choice
The %Trace% variable could simply be REM
, which will behave like @REM
so long as you have Echo Off
; however, if you have Echo On
, it results in printed REM lines for the no trace option (trace = 0). For this reason, @REM
is probably preferred.
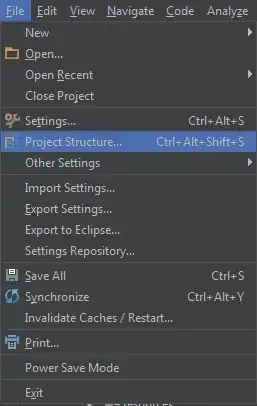
Versus this...

Alternatives to @REM
are <linefeed>
or ::
to mask the entire line and produce the following output even with Echo On
and trace = 0; however, both of these options are problematic inside of bracketed code segments. The only benefit I can think of for them is that they might be faster, but that should be negligent.
<linefeed>
is a non-obvious choice and works because the parser stops parsing and goes to the next line whenever it encounters a linefeed character. To set a variable equal to linefeed is a little tricky, but can be done as follows...
if %trace% EQU 1 (
set trace=
) else (
set trace=
)
::The two new lines after "trace=" are required
See this answer as to why two lines are needed.