You can use the Alert class with the ERROR alert type.
Below is a working example:
MyApplication.java
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// do something
throw new RuntimeException();
} catch (Exception ex) {
displayApplicationError();
}
}
private void displayApplicationError() {
Platform.runLater(() -> {
new ApplicationError().start(new Stage());
Platform.exit();
});
}
ApplicationError.java
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage stage) {
Alert alert = new Alert(AlertType.ERROR);
alert.setTitle("ERROR");
alert.setHeaderText(null);
alert.setContentText("Application has Stopped Working");
alert.showAndWait();
}
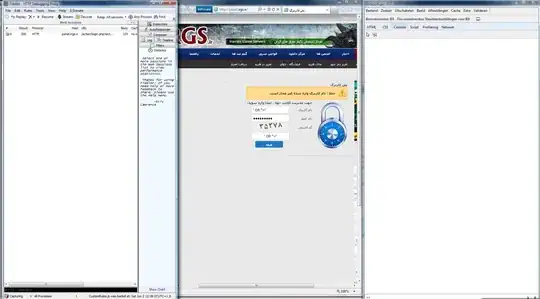
The alert will have a native look and feel on Windows, you can find more examples here.