You can declare an associative array with the keys as the node property with their values, then use a function to set your theme:
var primaryColor = document.documentElement.style.getPropertyValue('--primaryColor');
var secondaryColor = document.documentElement.style.getPropertyValue('--secondaryColor');
var errorColor = document.documentElement.style.getPropertyValue('--errorColor');
var themeColors = {}
themeColors["--primaryColor"] = primaryColor;
themeColors["--secondaryColor"] = secondaryColor;
themeColors["--errorColor"] = errorColor;
function setTheme(theme) {
for (key in theme) {
let color = theme[key];
document.documentElement.style.setProperty(key, color);
}
}
A working example I used with Atom and Bootstrap:
var backgroundColor = document.documentElement.style.getPropertyValue('--blue');
backgroundColor = "#dc3545";
function setTheme(theme) {
for (key in theme) {
let color = theme[key];
document.documentElement.style.setProperty(key, color);
}
}
var theme = {}
theme["--blue"] = backgroundColor;
setTheme(theme);
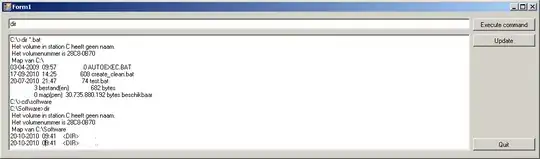
>>Edit<<
Nadeem clarified the question a bit better with a comment below, unfortunately however I've learned that :root
can be accessed with get Window.getComputedStyle()
however this doesn't return CSS Variable declarations.
A hack around this is just to read the css file, parse it for variables and stuff them into an associative array, but even this assumes you know where to get that css file...
//an associative array that will hold our values
var cssVars = {};
var request = new XMLHttpRequest();
request.open('GET', './css/style.css', true);
request.onload = function() {
if (request.status >= 200 && request.status < 400) {
//Get all CSS Variables in the document
var matches = request.responseText.match(/(--)\w.+;/gi);
//Get all CSS Variables in the document
for(let match in matches) {
var property = matches[match];
//split the Variable name from its value
let splitprop = property.split(":")
//turn the value into a string
let value = splitprop[1].toString()
cssVars[splitprop[0]] = value.slice(0, -1); //remove ;
}
// console.log(cssVars);
// > Object {--primaryColor: "aliceblue", --secondaryColor: "blue", --errorColor: "#cc2511"}
// console.log(Object.keys(cssVars));
// > ["--primaryColor", "--secondaryColor", "--errorColor" ]
setTheme(cssVars)
} else {
// We reached our target server, but it returned an error
}
};
request.onerror = function() {
console.log("There was a connection error");
};
request.send();
function setTheme(theme) {
var keys = Object.keys(theme)
for (key in keys) {
let prop = keys[key]
let color = theme[keys[key]];
console.log(prop, color);
// --primaryColor aliceblue etc...
}
}