This is not a python specific problem. I had this problem also with Node.js
. The problem is that essentially when the subscription errors it falls over and never receives a message again. BTW it should not do this and this is a bug.
It took me 3 days to find the fix but its simple! Its documented on github. Essentially, you have 2 or 3 options.
(1) Use grpc (whatever that is)
Here's the node code (simple enough to translate to your coding language):
const {PubSub} = require('@google-cloud/pubsub');
const grpc = require('grpc');
const pubsub = new PubSub({grpc});
This is the recommended approach with the downside that the grpc package is now deprecated (by grpc
themselves not by Google). There is a package called @grpc/grpc-js
that has replaced grpc
but I've no idea how to use it in conjunction with @google-cloud/pubsub
. Using grpc
is the solution I used and I can vouch it works! My subscription keeps receiving messages now even after it errors!
OR alternatively
(2) Re-init PubSub subscription on an error
If you are facing this problem, then just re-initialise the subscription on an error:
const initSubscriber = () => {
const pubsub = new PubSub();
const subscription = pubsub.subscription(topic, options);
subscription.on('message', handler.handleMessage);
subscription.on('error', e => {
initSubscriber();
});
};
initSubscriber();
Whilst this approach is reported to work, when the aforementioned bug no longer exists, then this approach may cause problems / have side effects. I cannot vouch for this approach as I've never tried it. If you are desperate give it a go.
(3) Increase acknowledgement deadline
This is not so much a fix as a potential workaround for some scenarios. In my experience, the subscription errors when the message is ack'd after the acknowledgement deadline. By increasing the acknowledgement deadline, you reduce the chance of this happening and thus the subscription won't error and thus the subscription won't fall over and thus you won't have a problem to fix! Obviously, if the subscription did ever fall over then you would be in trouble and solutions (1) and (2) still apply.
(4) General tips
The advice given in @KamalAboul-Hosn answer is useful and may apply to your case. It did not help for me but may help for some.
(5) Bonus tip
In Google Cloud Platform > Pub/Sub > Subscriptions
You can see how many messages have not yet been acknowledged. If acknowledgements are not happening (the unacked message count shown in the graph does not decrease) AFTER your subscription errors THEN you know this solution is the right one for you!
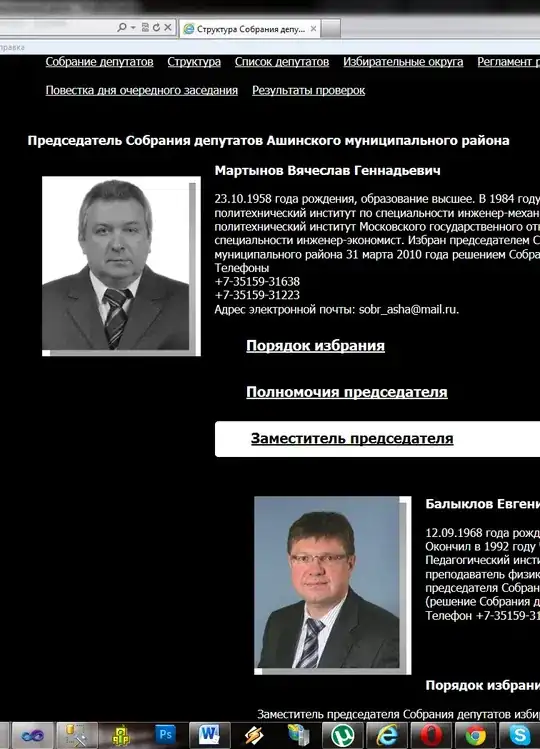
I took the time to write this up to save you 3 days coz J-E^S^-U-S died and rose 3 days later to save your life. He loves you and wanted you to know that :D