Updating my answer, Do Yo want something like this :
import java.util.Random;
import java.util.Scanner;
public class randomLetters extends Thread {
String con = "bcdfghjklmnpqrstvwxyz"; // constant
String vow = "aeiouy"; // Vowel
private static Scanner input = new Scanner(System.in);
char[] option = new char[10]; // total characters available to player
String word; // answer given by player after 2 minute
static int score = 0; // player score
static int round = 1; // round number
Boolean startPlaing = true;
@Override
public void run() { // run method of thread
// ---------------------------------------------------->
// game loop
gameLoop();
// ---------------------------------------------------->
}
public static void main(String args[]) { // Main function
new randomLetters().start(); // start method calls run()
}
// ---------------------------------------------------->
// calculating score : change the score logic as per your game rule
public void getScore(String word) {
// using rule : Words are worth one point per letter, but a nine-letter
// word is worth double; that is, 18 points.
score = word.length();
if (word.length() >= 9)
score = 18;
}
// ---------------------------------------------------->
// ---------------------------------------------------->
private void gameLoop() {
while (startPlaing) {
System.out
.println("Round : "
+ round
+ " Score : "
+ score
+ "\n---------------------------------------------------->");
for (int i = 0; i < 10; i++) { // Prompt the user to chose a vowel
// of a
// consonant
System.out
.println("> Enter (V) for vowel and (C for consonnant)");
String V_or_C = input.next();
if (V_or_C.equalsIgnoreCase("V")) { // generating a random vowel
option[i] = vow.charAt(new Random().nextInt(vow.length()));
System.out.println(option[i]);
}
if (V_or_C.equalsIgnoreCase("C")) { // generating a random
// constant
option[i] = con.charAt(new Random().nextInt(con.length()));
System.out.println(option[i]);
}
}
startPlaing = false;
try {
System.out.print("\nAvailable character : ");
for (char i : option) { // printing the selected options
System.out.print(" [ " + i + " ]");
}
System.out.println("\n\nGame waiting for 2 minute");
Thread.sleep(120000); // 1000 milliseconds = 1 seconds 120
// seconds = 2 minute
System.out.println("\n Time up !! Enter your word :");
word = input.next();
if (check(word)) {
System.out.println("\nStrart next round (y/n): ");
String choice = input.next();
if (choice.equalsIgnoreCase("y")) {
System.out
.println("\nLets Start---------------------------------------------------->\n");
round += 1; // increment round
startPlaing = true;
} else if (choice.equalsIgnoreCase("n")) {
startPlaing = false;
System.out
.println("\nGood Bye :\nYour score till now :"
+ score);
}
}
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// ---------------------------------------------------->
// ----------------------Start of check
// function----------------------------->
// check function validate game rules like:
// 1. word or answer is not empty
// 2. word is in dictionary
// 3. word should only comprise using characters available as option
// add rules as per your understanding of game.
public Boolean check(String s) {
String[] dictionary = { "help", "permited", "elephant", "relationship",
"ab", "cv", "az", "a", "v", "zzz", "aeiou", "aeioucv",
"aeiouz", "aeio", "aeiouaeio", "aei", "oye" };
// add
// valid
// words
// to
// this
// dictionary
System.out.println("\nYou entered : " + s);
if (s.length() != 0) { // if user has entered something
char[] word = s.toCharArray();
// check if answer only include character available as option
// ---START
Boolean b = false;
for (char a : word) {
for (int j = 0; j < option.length; j++) {
/*
* System.out.println("matching :" + a + " with " +
* option[j] + " " + (a != option[j]));
*/// uncomment to see the comparison
if (a != option[j]) {
b = true;
} else {
b = false;
break;
}
}
if (b) {
System.out
.println("\n Wrong answer (Game exit) : Your answer include characters other than option available to you . \n Your score till now is : "
+ score);
return false;
}
}
// check if answer only include character available as option ---END
// --------------------START------------------------------->
//
// add your code here for rule saying only same number of character
// should be used
// example: if there is only one 'c' available in option then player
// answer can have only one 'c'
//
// --------------------END------------------------------->
// check if answer is a valid word from words available in
// dictionary ---START
boolean notInDictionary = true;
for (String c : dictionary) {
/*
* System.out.println("matching :" + s + " with " + c + " " +
* c.equals(s));
*/// uncomment to see the matching
if (c.equals(s)) // if word is in dictionary
{
notInDictionary = false;
getScore(c);
break;
}
}
if (notInDictionary) {
// enter your logic for this situation
// Example rule that you can add :If a contestant tries a longer
// word that is not in the programme's dictionaries, his or her
// word is rejected, but his or her opponent may score the
// points for its length by giving a shorter, valid word.
System.out
.println("\nYour answer is not a valid word available in our dictionary \nscore :"
+ score);
return false;
}
// check if answer is a valid word from words available in
// dictionary ---END
return true;
}
// ---------------------------------------------------->
// If no input is entered by user
if (s.length() == 0) {
System.out.println("\nGame Exit as No input and your score is : "
+ score);
return false;
}
// ---------------------------------------------------->
return false;
}
// -------------------End of check------------------------------->
}
OUTPUT:
1.Take choice and generate 10 option.
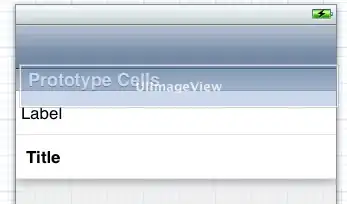
- If user enter wrong input (That is if user uses characters other then available to him as option).
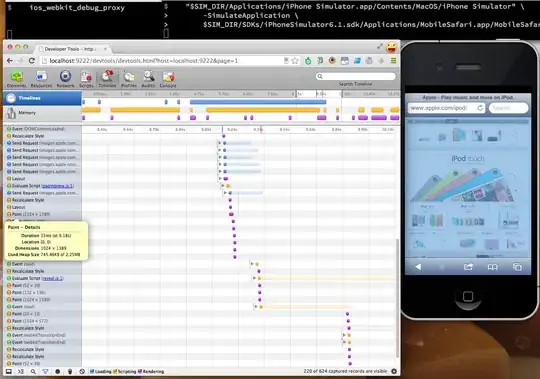
- If user given answer exist in the Dictionary of words available to us then calculate the score using the rule :
Words are worth one point per letter, but a nine-letter word is worth double that is, 18 points.

- If player chose to continue playing go to next round and if player chose 'n'.
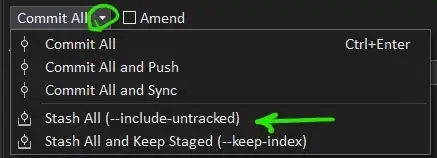
Game reference was taken from here
I have provided comments within the code so that you can update the game as per your requirements.
I hope this answer is close to what you were looking for :)
you can comment if any update is required.