Just for completeness, here's a variant that uses print()
(works on Python 2.6-3.x):
from __future__ import print_function
try: from cStringIO import StringIO
except ImportError:
from io import StringIO
def to_int(nums, _s = StringIO()):
print(*nums, sep='', end='', file=_s)
s = _s.getvalue()
_s.truncate(0)
return int(s)
Time performance of different solutions
I've measured performance of @cdleary's functions. The results are slightly different.
Each function tested with the input list generated by:
def randrange1_10(digit_count): # same as @cdleary
return [random.randrange(1, 10) for i in xrange(digit_count)]
You may supply your own function via --sequence-creator=yourmodule.yourfunction
command-line argument (see below).
The fastest functions for a given number of integers in a list (len(nums) == digit_count
) are:
len(nums)
in 1..30
def _accumulator(nums):
tot = 0
for num in nums:
tot *= 10
tot += num
return tot
len(nums)
in 30..1000
def _map(nums):
return int(''.join(map(str, nums)))
def _imap(nums):
return int(''.join(imap(str, nums)))
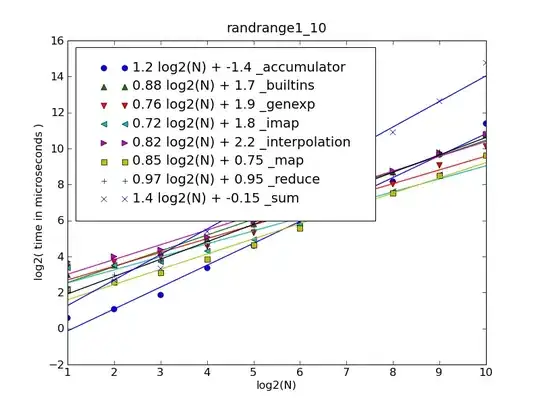
|------------------------------+-------------------|
| Fitting polynom | Function |
|------------------------------+-------------------|
| 1.00 log2(N) + 1.25e-015 | N |
| 2.00 log2(N) + 5.31e-018 | N*N |
| 1.19 log2(N) + 1.116 | N*log2(N) |
| 1.37 log2(N) + 2.232 | N*log2(N)*log2(N) |
|------------------------------+-------------------|
| 1.21 log2(N) + 0.063 | _interpolation |
| 1.24 log2(N) - 0.610 | _genexp |
| 1.25 log2(N) - 0.968 | _imap |
| 1.30 log2(N) - 1.917 | _map |
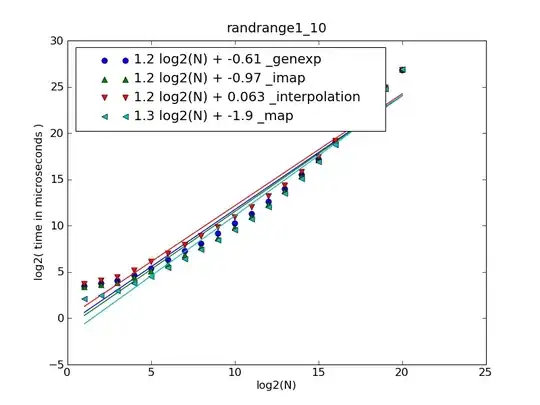
To plot the first figure download cdleary.py
and make-figures.py
and run (numpy
and matplotlib
must be installed to plot):
$ python cdleary.py
Or
$ python make-figures.py --sort-function=cdleary._map \
> --sort-function=cdleary._imap \
> --sort-function=cdleary._interpolation \
> --sort-function=cdleary._genexp --sort-function=cdleary._sum \
> --sort-function=cdleary._reduce --sort-function=cdleary._builtins \
> --sort-function=cdleary._accumulator \
> --sequence-creator=cdleary.randrange1_10 --maxn=1000