If I understand well you want to have two buttons (Next, Back)
I make a little project and I will be happy if it helped you.
If you want this, continue reading:
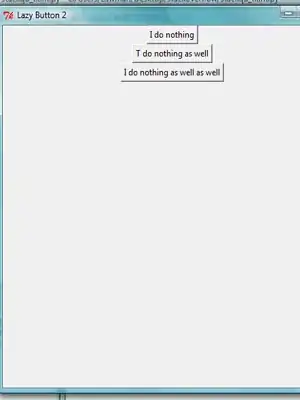
First we have to declare imageCount
and a List<string>
: Imagefiles
so we have this
List<string> Imagefiles = new List<string>();
int imageCount = 0;
imageCount
helps to change images using buttons
Imagefiles
contains all Image paths of our photos
Now to change photos we have to declare a path first which wil contains all photos.
I use FolderBrowserDialog
using (var fbd = new FolderBrowserDialog())
{
DialogResult result = fbd.ShowDialog();
if (result == DialogResult.OK && !string.IsNullOrWhiteSpace(fbd.SelectedPath))
{
findImagesInDirectory(fbd.SelectedPath);
}
}
You will see that I use findImagesInDirectory
and this method doesnt exist. We have to create it.
This method will help us to filter all the files from the path and get only the image files
private void findImagesInDirectory(string path)
{
string[] files = Directory.GetFiles(path);
foreach(string s in files)
{
if (s.EndsWith(".jpg") || s.EndsWith(".png")) //add more format files here
{
Imagefiles.Add(s);
}
}
try
{
pictureBox1.ImageLocation = Imagefiles.First();
}
catch { MessageBox.Show("No files found!"); }
}
I use try
because if no image files, with the above extension, exists code will break.
Now we declare all Image files (if they exists)
Next Button
private void nextImageBtn_Click(object sender, EventArgs e)
{
if (imageCount + 1 == Imagefiles.Count)
{
MessageBox.Show("No Other Images!");
}
else
{
string nextImage = Imagefiles[imageCount + 1];
pictureBox1.ImageLocation = nextImage;
imageCount += 1;
}
}
Previous Button
private void prevImageBtn_Click(object sender, EventArgs e)
{
if(imageCount == 0)
{
MessageBox.Show("No Other Images!");
}
else
{
string prevImage = Imagefiles[imageCount -1];
pictureBox1.ImageLocation = prevImage;
imageCount -= 1;
}
}
I believe my code help. Hope no bugs!