Depending on your implementation, one of the below methods should work for you.
self.navigationItem.title = "title"
or
self.navigationBar.topItem?.title = "title"
If you are using a custom tabbar made with UIButtons and container
view, then add this to the button action or if you are using a native
UITabBarController, then set it's delegate to self and call this on
the didSelectViewController delegate method of the UITabBarController.
.
EDIT
After seeing your code, you need to use this property :
self.tabBarController?.navigationItem.title = "Profile"
and call this in every view controller's viewWillAppear, example for ProfileViewController
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
self.tabBarController?.navigationItem.title = "Profile"
}
Also, make sure that in storyboard, you set the view controller's class to the respective code class like :
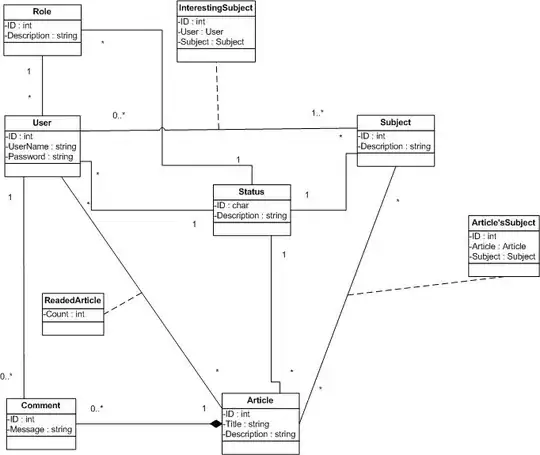
and remove the text from the custom navigation bar you used:
