This is more like a hack than a direct conversion: but it should give the equivalent result:
Basically:
- Save the image to a stream
- Rewind the stream
- Tell the Wpf image to use the stream as its stream source
Here is a class that does that:
using System;
using System.Collections.Generic;
using System.Drawing.Imaging;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Media;
using System.Windows.Media.Imaging;
namespace StackCsWpf
{
public class ImageUtils
{
public static ImageSource ToImageSource(System.Drawing.Image image, ImageFormat imageFormat)
{
BitmapImage bitmap = new BitmapImage();
using (MemoryStream stream = new MemoryStream())
{
// Save to the stream
image.Save(stream, imageFormat);
// Rewind the stream
stream.Seek(0, SeekOrigin.Begin);
// Tell the WPF BitmapImage to use this stream
bitmap.BeginInit();
bitmap.StreamSource = stream;
bitmap.CacheOption = BitmapCacheOption.OnLoad;
bitmap.EndInit();
}
return bitmap;
}
}
}
Now as an illustration I can use the method above to display the image in a Wpf Image component.
/// <summary>
/// Logique d'interaction pour MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
System.Drawing.Image winFormImg = System.Drawing.Image.FromFile("leaves.jpg");
Image1.Source = ImageUtils.ToImageSource(winFormImg, ImageFormat.Jpeg);
}
}
Image1 is a simple Wpf Image component that I dragged from the toolbox into my Wpf App main window's grid.
It renders nicely:
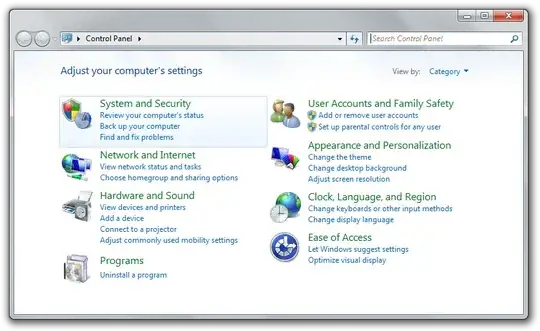
Ref: Msdn forums