This suggestion will work for any type of index values (string, dates or integers) as long as they are unique.
Short Answer:
Create a pandas dataframe of the longest series. This dataframe will have an index. Get the last 50 index values from that series and associate it with your prediction values in a new dataframe. Your two dataframes will have different lenghts, so you'll have to merge
them together in order to get two series of equal lengths. With this approach, your first 100 values of your prediction values will be empty, but your data will have an associated index so that you can plot it against your test1 series.
The details:
Since you did not share a reproducible dataset, I made some random data that should match the structure of your dataset. The first snippet below will reproduce your situation and make two arrays test1 and **predictions1 **available for a suggested solution.
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
np.random.seed(123456)
rows = 150
df = pd.DataFrame(np.random.randint(-4,5,size=(rows, 1)), columns=['test1'])
datelist = pd.date_range(pd.datetime(2017, 1, 1).strftime('%Y-%m-%d'), periods=rows).tolist()
df['dates'] = datelist
df = df.set_index(['dates'])
df.index = pd.to_datetime(df.index)
df['test1'] = df['test1'].cumsum()
# Get the last 50 values of test1 (as array instead of dataframe)
# to mimic the names and data types of your source data
test1 = df['test1'].values
predicionts1 = df['test1'].tail(50).values
predictions1 = predicionts1*1.1
# Reproducing your situation:
import matplotlib.pyplot as plt
plt.figure(figsize=(15,8))
plt.plot(test1)
plt.plot(predictions1, color = 'red')
plt.show()
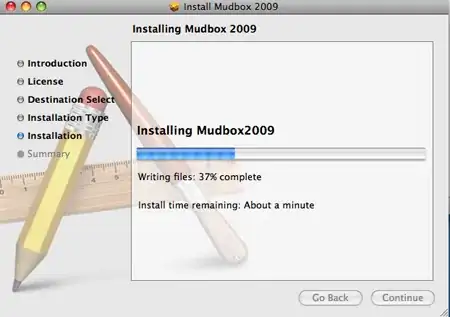
The following snippet will superimpose predictions1 on test1:
# Make a new dataframe of your prediction values
df_new = pd.DataFrame(test1)
df_new.columns = ['test1']
# Retrieve index values
new_index = df_new['test1'].tail(len(predictions1)).index
# Make a dataframe with your prediction values and your index
new_series = pd.DataFrame(index = new_index, data = predictions1)
# Merge the dataframes
df_new = pd.merge(df_new, new_series, how = 'left', left_index=True, right_index=True)
df_new.columns = ['test1', 'predictions1']
# And plot it
import matplotlib.pyplot as plt
plt.figure(figsize=(15,8))
plt.plot(df_new['test1'])
plt.plot(df_new['predictions1'], color = 'red')
plt.show()
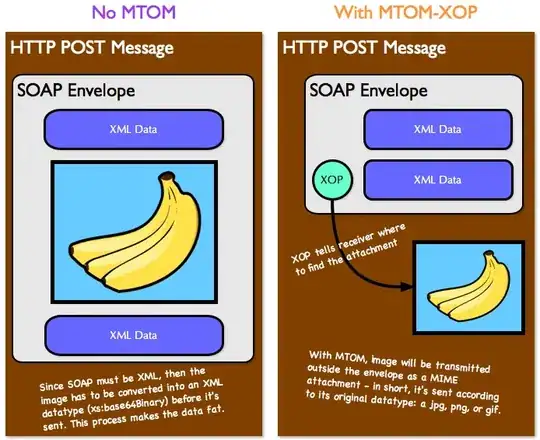