You need custom bar BarChartRenderer to achieve this. I have provided a rough sample. Hope it helps.
Code for setting the barchart
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// custom colors that you want on top of the bars
ArrayList<Integer> myColors = new ArrayList<>();
myColors.add(Color.BLACK);
myColors.add(Color.YELLOW);
myColors.add(Color.BLUE);
myColors.add(Color.DKGRAY);
myColors.add(Color.GREEN);
myColors.add(Color.GRAY);
String[] myText = {"A Round", "B Round", "C Round", "D Round", "E Round", "F Round"};
BarChart mChart = (BarChart) findViewById(R.id.barChart);
mChart.setDrawBarShadow(false);
mChart.getDescription().setEnabled(false);
mChart.setDrawGridBackground(false);
XAxis xaxis = mChart.getXAxis();
xaxis.setDrawGridLines(false);
xaxis.setPosition(XAxis.XAxisPosition.BOTTOM);
xaxis.setDrawLabels(true);
xaxis.setDrawAxisLine(false);
YAxis yAxisLeft = mChart.getAxisLeft();
yAxisLeft.setPosition(YAxis.YAxisLabelPosition.INSIDE_CHART);
yAxisLeft.setDrawGridLines(false);
yAxisLeft.setDrawAxisLine(false);
yAxisLeft.setEnabled(false);
mChart.getAxisRight().setEnabled(false);
// set your custom renderer
mChart.setRenderer(new BarChartCustomRenderer(mChart, mChart.getAnimator(), mChart.getViewPortHandler(), myColors));
mChart.setDrawValueAboveBar(true);
Legend legend = mChart.getLegend();
legend.setEnabled(false);
ArrayList<BarEntry> valueSet1 = new ArrayList<BarEntry>();
for (int i = 0; i < 6; ++i) {
BarEntry entry = new BarEntry(i, (i + 1) * 10);
valueSet1.add(entry);
}
List<IBarDataSet> dataSets = new ArrayList<>();
BarDataSet barDataSet = new BarDataSet(valueSet1, " ");
barDataSet.setValueFormatter(new MyFormatter(myText));
barDataSet.setColor(Color.CYAN);
dataSets.add(barDataSet);
BarData data = new BarData(dataSets);
mChart.setData(data);
}
public class MyFormatter implements IValueFormatter {
String[] text;
public MyFormatter(String[] text) {
this.text = text;
}
@Override
public String getFormattedValue(float value, Entry entry, int dataSetIndex, ViewPortHandler viewPortHandler) {
return String.valueOf((int)value)+"M" + ", " + text[(int) entry.getX()];
}
}
}
Custom Renderer
public class BarChartCustomRenderer extends BarChartRenderer {
private Paint myPaint;
private ArrayList<Integer> myColors;
public BarChartCustomRenderer(BarDataProvider chart, ChartAnimator animator, ViewPortHandler viewPortHandler, ArrayList<Integer> myColors) {
super(chart, animator, viewPortHandler);
this.myPaint = new Paint();
this.myColors = myColors;
}
@Override
public void drawValues(Canvas c) {
super.drawValues(c);
// you can modify the original method
// so that everything is drawn on the canvas inside a single loop
// also you can add logic here to meet your requirements
int colorIndex = 0;
for (int i = 0; i < mChart.getBarData().getDataSetCount(); i++) {
BarBuffer buffer = mBarBuffers[i];
float left, right, top, bottom;
for (int j = 0; j < buffer.buffer.length * mAnimator.getPhaseX(); j += 4) {
myPaint.setColor(myColors.get(colorIndex++));
left = buffer.buffer[j];
right = buffer.buffer[j + 2];
top = buffer.buffer[j + 1];
bottom = buffer.buffer[j + 3];
// myPaint.setShader(new LinearGradient(left,top,right,bottom, Color.CYAN, myColors.get(colorIndex++), Shader.TileMode.MIRROR ));
c.drawRect(left, top, right, top+5f, myPaint);
}
}
}
@Override
public void drawValue(Canvas c, IValueFormatter formatter, float value, Entry entry, int dataSetIndex, float x, float y, int color) {
String text = formatter.getFormattedValue(value, entry, dataSetIndex, mViewPortHandler);
String[] splitText;
if(text.contains(",")){
splitText = text.split(",");
Paint paintStyleOne = new Paint(mValuePaint);
Paint paintStyleTwo = new Paint(mValuePaint);
paintStyleOne.setColor(Color.BLACK);
paintStyleTwo.setColor(Color.BLUE);
c.drawText(splitText[0], x, y-20f, paintStyleOne);
c.drawText(splitText[1], x, y, paintStyleTwo);
}
//else{
// super.drawValue(c, formatter, value, entry, dataSetIndex, x, y, color);
//}
}
}
RESULT
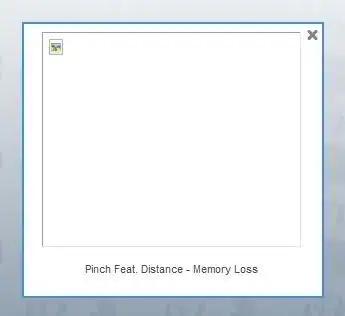
you can also do a gradient effect for the entire bar by slightly modifying the custom renderer :
myPaint.setShader(new LinearGradient(left,top,right,bottom, Color.CYAN, myColors.get(colorIndex++), Shader.TileMode.MIRROR ));
c.drawRect(left, top, right, bottom, myPaint);
you can similarly draw and style your text using the custom renderer.
Check this to learn more about custom renderers.
Update for using drawables instead of colors
//get bitmap from a drawable
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.myDrawable);
after than you can create a list of bitmaps and pass in onto the renderer instead of the list of colors.
if you want to draw on just the top of the bar you can use this :
c.drawBitmap(bitmap.get(index++), null, new RectF(left, top, right, top+5f), null);
or if you want to cover the entire bar, you can do so by using the bitmap like this:
c.drawBitmap(bitmap.get(index++), null, new RectF(left, top, right, bottom), null);