Congratulations, you've fallen victim to a number of conspiring issues.
The main culprit is FlowLayout
, which is the default layout manager for JPanel
. Essentially, when you add your, rather large, JTextArea
to the panel, the FlowLayout
is trying to honour the preferred size as best as it can within the constraints of the available space. For reasons I'm not 100% sure of, that means laying out the component beyond the visible bounds of the container.
If you type enough text, you will begin to see it.
While there are a number of ways you might fix this, they are basically the same solution - use a different layout manager.
For this example, I've just used a BorderLayout
instead
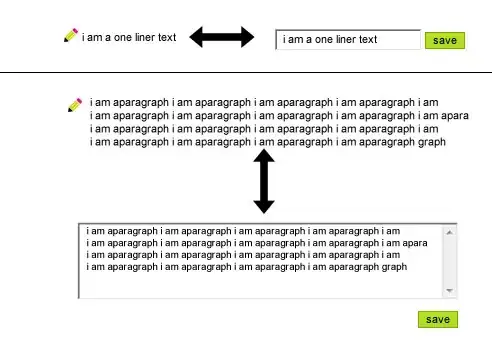
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSplitPane;
import javax.swing.JTextArea;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class Test {
public static void main(String[] args) {
new Test();
}
private JPanel jp1, jp2;
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
jp1 = new JPanel();
jp2 = new JPanel(new BorderLayout());
JLabel label = new JLabel("text");
JTextArea ta = new JTextArea(50, 50);
ta.setText("some text");
jp2.add(label, BorderLayout.NORTH);
jp2.add(new JScrollPane(ta));
JSplitPane jsp = new JSplitPane(JSplitPane.HORIZONTAL_SPLIT, jp1, jp2);
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(jsp);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
}