After reading the helfpful resources from the comments, here is my approach how to load the debug redistributable of VS2008 (msvcr90d.dll) in VS2015 (release and debug).
First your application needs a manifest file. What is a manifest file? This page summarizes it pretty well: http://www.samlogic.net/articles/manifest.htm
A manifest is a XML file that contains settings that informs Windows
how to handle a program when it is started. The manifest can be
embedded inside the program file (as a resource) or it can be located
in a separate external XML file.
A manifest file can be placed next to our executable OR is embedded (default). To switch/change it go to Projects Property Page -> Linker -> Manifest Tool -> Input and Output -> Embed Manifest
.
The pragma in the source below adds a few lines to our (by default) embedded manifest file into our executable.
Go to C:\Program Files (x86)\Microsoft Visual Studio 9.0\VC\redist\Debug_NonRedist\amd64\Microsoft.VC90.DebugCRT
and copy all files next to your executable
Open the new Microsoft.VC90.DebugCRT.manifest
and remove the token publicKeyToken="1fc8b3b9a1e18e3b"
. If it is inside, Windows keeps rejecting to load the msvcr90d.dll. Please don't ask me why.
Compile and Execute your program
#include <Windows.h>
#pragma comment(linker,"/manifestdependency:\"type='win32' "\
"name='Microsoft.VC90.DebugCRT' "\
"version='9.0.21022.8' "\
"processorArchitecture='amd64' "\
"\"")
int main()
{
auto* lib = LoadLibrary(L"msvcr90d.dll");
if (!lib)
{
return -1;
}
FreeLibrary(lib);
return 0;
}
Background
Why do we have an external manifest file (Microsoft.VC90.DebugCRT.manifest), and an embedded one (through the pragma) for this solution? It looks like the pragma only offers a limited amount of options how your manifest can look like. One option is to reference to another manifest file which contains more information.

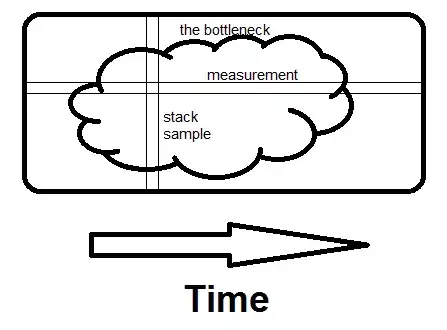
Edit: The answers in this MSDN enter link description here thread also helped a lot.
Edit 2: Instead of referencing from the embedded manifest to the external Microsoft.VC90.DebugCRT.manifest
, you could already embed this one to your executable (can also be done by the Property Pages), but I came to this conclusion just too late but my initial solution hopefully gives some more insights