Does the pointer size matter?
No, the size of the pointer doesn't matter. What matter is the type of pointer, i.e. the type it points to.
The number of bytes copied when assigning through a pointer depends on the pointer type. If the pointer type is a char pointer, it will copy sizeof(char)
bytes. If the pointer type is an int pointer, it will copy sizeof(int)
bytes.
Why does this code print 10 = -246 instead of 10 = 10?
It's system dependent. Since you get this result, you are probably on a little endian system which means that data in memory is stored with LSB first (i.e. a pointer to a variable points to LSB of that variable).
So what happens in your code is that LSB of variable i
is copied to LSB of variable j
. Since sizeof(int)
is more than 1, you'll not end in a situation where i
and j
are equal. Simply because you didn't copy all bytes of i
into j
.
Assuming a 32 bit int
it may look like:
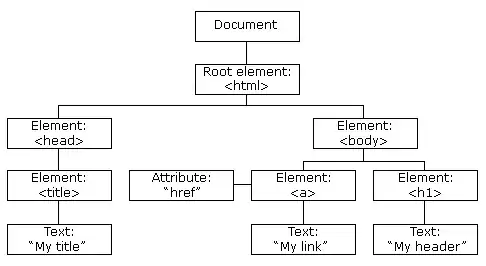