You can use ipgeolocation which is a free IP API that provides country, city, state, province, latitude, longitude and etc.
let apiKey = "your_api_key_here";
async function getLocation (ip) {
let url = `https://api.ipgeolocation.io/ipgeo?apiKey=${apiKey}&ip=${ip}`;
await fetch(url).then((response) =>
response.json().then((json) => {
const output = `
---------------------
Country: ${json.country_name}
State: ${json.state_prov}
City: ${json.city}
District: ${json.district}
Lat / Long: (${json.latitude}, ${json.longitude})
---------------------
`;
console.log(output);
})
);
};
getLocation('154.28.188.208') // prints the location
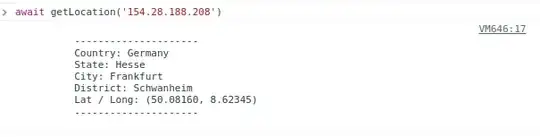