I learnt that gl coordinate system value lies between -1 to +1. But this program is working nicely. How it is possible?
Of course, the normalized device space is in range [-1, 1] and the normalized device space is mapped to the viewport.
But you have tdefined a persepctive projection matrix (see gluPerspective
):
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(65.0, (GLfloat) w/(GLfloat) h, 1.0, 20.0);
The projection matrix describes the mapping from 3D points of a scene, to 2D points of the viewport. It transforms from eye space to the clip space, and the coordinates in the clip space are transformed to the normalized device coordinates (NDC) by dividing with the w
component of the clip coordinates. The NDC are in range (-1,-1,-1) to (1,1,1).
At Perspective Projection the projection matrix describes the mapping from 3D points in the world as they are seen from of a pinhole camera, to 2D points of the viewport.
The eye space coordinates in the camera frustum (a truncated pyramid) are mapped to a cube (the normalized device coordinates).
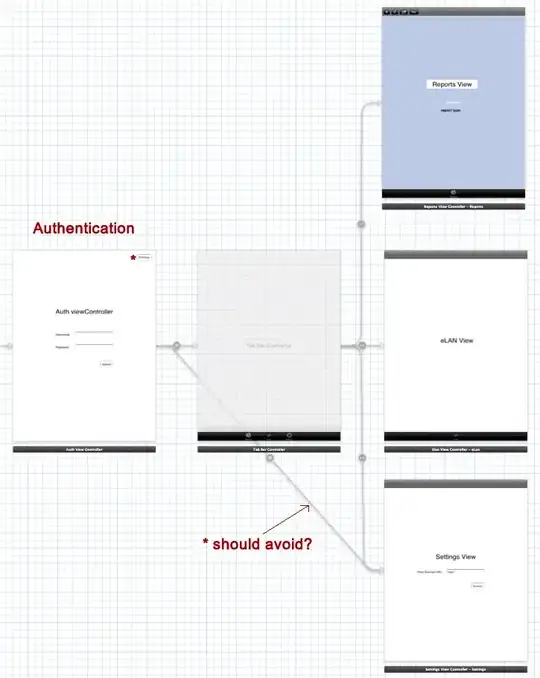
Further the letters are scaled by glScalef
:
glScalef(scale, scale, scale);
glScalef
scales the size of the geometry. The parameters specify the scale factors along the x
, y
, and z
axes. In your case all 3 parameters are 0.003, so the letters are reduced to 0.3% of the size as would it be without scaling.
glTranslatef
moves the letters in its position. The paramters specify the x
, y,
and z
coordinates of a translation. vector.
When you set up the projection matrix, then the distance to the near and far plane is set to 1.0 and 20.0. Since the letters have to be in between the near and the far plane, they are shift to this range by the translation along the z-axis.
glTranslatef(0.0, 0.0, -5.0);
The position in the xy-plane of the viewport is defined by the 2nd translation:
glTranslatef(-4.0, 2.5, 0.0);
See also OpenGL translation before and after a rotation for some more explanations about the OpenGLs fixed function pipeline matrix stack.
Extension to the answer regarding to the comment
I am beginner in OpenGL. I am sorry if my question is appropriate. glTranslatef(-4.0, 2.5, 0.0); In this how the parameters range is decided ? ( What is max and min value of x,y and z) . It moves the text to left or right. How can I calculate all these things?
Your perspective projection is defined:
gluPerspective(65.0, (GLfloat) w/(GLfloat) h, 1.0, 20.0);
At Perspective Projection the projection matrix describes the mapping from 3D points in the world as they are seen from of a pinhole camera, to 2D points of the viewport.
The minimum and maximum value for the z-coordianate are defined by the near and the far plane. In your case the near plane is 1.0 and the far plane is 20.0. Since the z-axis points out of the viewport, this means:
1.0 <= -z <= 20.0
The realtion between the projected area in view space and the Z coordinate of the view space is linear. It dpends on the field of view angle and the aspect ratio.
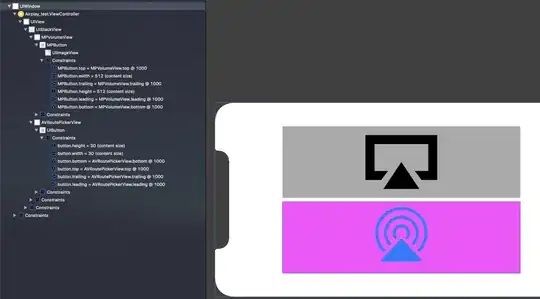
This means the size of the projected area on the viewport depends on the Z-distance to the view position and can be calculated like this:
a = w / h
t = tan(fov_y / 2) * 2
x_min = -z * a * -t/2
y_min = -z * -t/2
x_max = -z * a * t/2
y_max = -z * t/2
In your case:
w = 1024
h = 700
fov_y = 65°
z = -5.0
x_min = -4.66
y_min = -3.19
x_max = 4.66
y_max = 3.19
If the coordiante system of the view space is a Right-handed system, then the X-axis points to the left, the Y-axis up and the Z-axis out of the view (Note in a right hand system the Z-Axis is the cross product of the X-Axis and the Y-Axis).