For react-native versions greater than 0.56.0 the social share functionality is already implemented in the library, so extra libraries like react-native-share are no longer needed and they could be unmantained. In fact, I was using react-native-share library some months ago for older versions of react-native and I migrated the corresponding code to the react-native implementation that exports a Share class that has a share method and it's very easy to use.
Then, you can use share method to share data and react-native will know what apps have the phone installed. In the following image you can see how the sharing screen looks like in an Android phone with WhatsApp application installed:
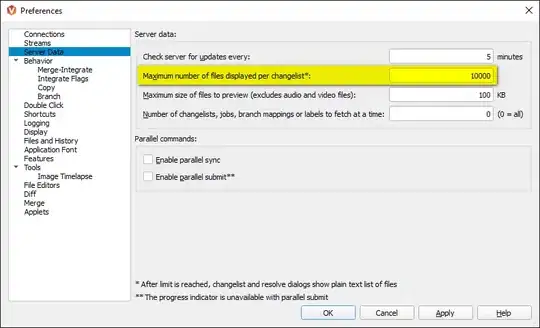
And this is how it would like in an iOS simulator with no app installed:
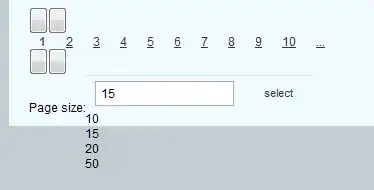
Here you can find an example of code:
import React, { Component } from 'react';
import {
Share,
Text,
TouchableOpacity
} from 'react-native';
const shareOptions = {
title: 'Title',
message: 'Message to share', // Note that according to the documentation at least one of "message" or "url" fields is required
url: 'www.example.com',
subject: 'Subject'
};
export default class ShareExample extends React.Component {
onSharePress = () => Share.share(shareOptions);
render(){
return(
<TouchableOpacity onPress={this.onSharePress} >
<Text>Share data</Text>
</TouchableOpacity>
);
}
}
Finally you have to options to send the image + text message:
- You can use the url field of the shareOptions adding the remote URI of the image so it can be previewed in the WhatsApp message, and the title or subject fields to add the text.
- You can share a base64 file url like this:
url: 'data:image/png;base64,<base64_data>'