You can do it at the command-line without any need for Python, using ImageMagick which is installed on most Linux distros and is available for macOS and Windows.
It will work for PNG, JPEG and around 200 other image types. Let's make a sample image:
convert xc:red xc:blue xc:lime +append \
\( xc:blue xc:red xc:lime +append \) \
\( xc:lime xc:red xc:blue +append \) \
-append start.png
And let's enlarge it so you can see it (I could have done it one go):
convert start.png -scale 400x start.png
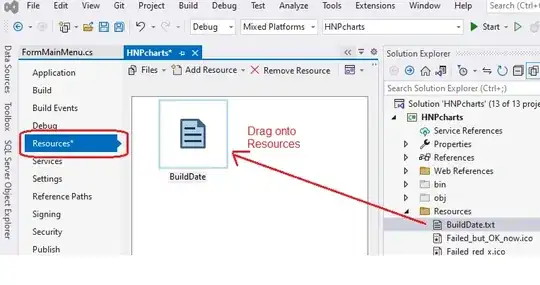
Now to your question, I can convert that to raw RGB (by interpreting all the image sizes, palettes, compression, and headers) and pass that through xxd
for hex formatting:
convert start.png -depth 8 rgb: | xxd -g3 -c9 -u
00000000: FF0000 0000FF 00FF00 .........
00000009: 0000FF FF0000 00FF00 .........
00000012: 00FF00 FF0000 0000FF .........
If you want to strip the address off the front and the dots off the end, you can use:
convert start.png -depth 8 rgb: | xxd -g3 -c9 -u | sed 's/ .*//; s/^.*: //'
FF0000 0000FF 00FF00
0000FF FF0000 00FF00
00FF00 FF0000 0000FF
The sed
command says... "Anywhere you see two spaces, remove them and all that follows. Remove anything up to and including a colon followed by a space.".
Or maybe plain dump is better:
convert start.png -depth 8 rgb: | xxd -g3 -c9 -u -p
FF00000000FF00FF00
0000FFFF000000FF00
00FF00FF00000000FF
Note: If using ImageMagick v7+, replace convert
with magick
in all examples above.