Sometimes setting a param as required
is not enough, because you don't only need that param to be present, but you need it in a specific format, there's an alternative using events
.
There are multiple steps, but once you're familiar with it, you'll do it very fast.
1) Create 2 new intents: Year - Confirmation
& Year - Confirmed
2) Add an event in the first intent: Intent > Events > ask-year
(or whatever name you like)
3) Add an output context: year-confirmation
4) Set a response asking the user to enter the year: Please provide the year...
5) Set parameter:
- Name: date
- Entity: -
- Value:
#ask-year.date
(This will come from event data, you will send it from your backend)
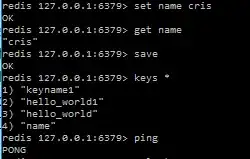
6) On your second intent Year - Confirmed
, add as input context: year-confirmation
(the output from the previous intent)
7) Set the same action as your main intent: insurance
8) Add some training phrases where you can match the year:
- 2017 (@sys.number:year)
- The year is @sys.number:year (Use template mode for this one)

Now you will have $year
as params.
9) Add one extra parameter:
- Name: date
- Entity: -
- Value:
#year-confirmation.date
(This will come from year-confirmation context)
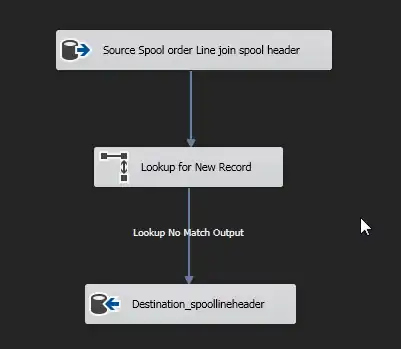
10) On your backend, when you receive the incompleted date you should send ask-year
event.
Node.js example, I don't know python
const apiai = require('apiai');
const client = apiai('my-dev-token');
function sendEvent(data) {
const request = client.eventRequest(data, {
sessionId: 'current-session'
});
request.on('response', response => {
// Push message to your UI
console.log(response.result.fulfillment.speech); // Please provide the year...
});
request.on('error', error => {
console.error('Event error: ', error);
});
request.end();
}
/* ... */
// Your insurance action handler
function insuranceHandler(result) {
const { parameters } = result;
if(parameters.date || parameters.date.includes('UUUU')) { // Or whatever check for invalid year
// Send previous date as data, so you will have it in the event response
return sendEvent({
name: 'ask-year',
data: {
date: parameters.date
}
});
}
// Year comes from `ask-year` intent
if(parameters.year)
parameters.date = parameters.date.replace('UUUU', parameters.year);
// Do whatever you need
}
Now when the date is incomplete, the event will be triggered, executing Year - Confirmation
and you will be asked to provide the year. After you provide one, Year - Confirmed
intent will be executed by your response. Now your backend will receive the insurance
action with an additional parameter, year