You could achieve this appearance by adding a -white background- UIView
on top the the table view and set its alpha
to the desired value.
At Storyboard, it would be similar to:

Note that at the document outline - views hierarchy the view is underneath the tableview, which means it would be on top of it.
Remark: it might be hard to add the view by dragging it directly to the view controller since it would be as subview in the table view; You could drag it in the document outline instead.
Then you could setup the desired constraints to it (I just added 0 leading, 0 trailing, 0 bottom and 70 height). Finally change its color opacity:
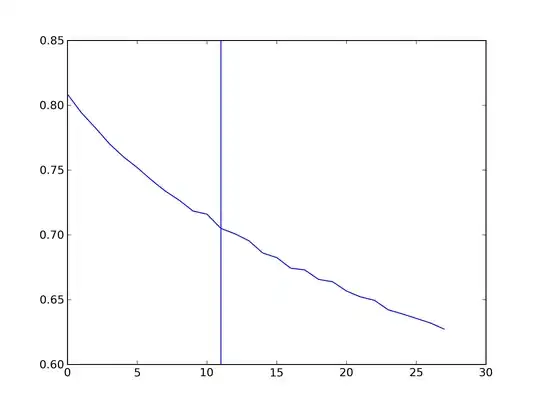
As you can see, I just edited the opacity to be 50% (alpha = 0.5
).
Furthermore:
you could also let the bottom view to has gradient of white and clear colors, it could even make it nicer! You might want to check:
How to Apply Gradient to background view of iOS Swift App