If the rest of the picture is one colour you can compare each pixel and find a different colour indicating the start of the picture like this please pay attention that I assume the top right hand corner to be the background colour, if this is not always the case, use a different approach (counting mode pixel colour for instance)!:
import numpy as np
from PIL import Image
import pprint
def get_y_top(pix, width, height, background, difference):
back_np = np.array(background)
for y in range(0, height):
for x in range(0, width):
if max(np.abs(np.array(pix[x, y]) - back_np)) > difference:
return y
def get_y_bot(pix, width, height, background, difference):
back_np = np.array(background)
for y in range(height-1, -1, -1):
for x in range(0, width):
if max(np.abs(np.array(pix[x, y]) - back_np)) > difference:
return y
def get_x_left(pix, width, height, background, difference):
back_np = np.array(background)
for x in range(0, width):
for y in range(0, height):
if max(np.abs(np.array(pix[x, y]) - back_np)) > difference:
return x
def get_x_right(pix, width, height, background, difference):
back_np = np.array(background)
for x in range(width-1, -1, -1):
for y in range(0, height):
if max(np.abs(np.array(pix[x, y]) - back_np)) > difference:
return x
img = Image.open('test.jpg')
width, height = img.size
pix = img.load()
background = pix[0,0]
difference = 20 #or whatever works for you here, use trial and error to establish this number
y_top = get_y_top(pix, width, height, background, difference)
y_bot = get_y_bot(pix, width, height, background, difference)
x_left = get_x_left(pix, width, height, background, difference)
x_right = get_x_right(pix, width, height, background, difference)
Using this information you can crop your image and save:
img = img.crop((x_left,y_top,x_right,y_bot))
img.save('test3.jpg')
Resulting in this:
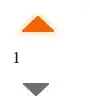