I know you already have an answer, but I thought I'd throw this out there. You could create an extension method that does this, which would simplify things a little when you want to use it (you can just type the number and then type .ToStringWithSpaces()
).
For example:
public static class Extensions
{
public static string ToStringWithSpaces(this int number)
{
var numStr = number.ToString();
var len = numStr.Length;
var result = new StringBuilder();
for (var i = 0; i < len; i++)
{
if (i > 0 && i % 3 == 0) result.Insert(0, " ");
result.Insert(0, numStr[len - 1 - i]);
}
return result.ToString();
}
}
Then, in use:
private static void Main()
{
var bigNumber = int.MaxValue;
Console.WriteLine(123.ToStringWithSpaces());
Console.WriteLine(1234.ToStringWithSpaces());
Console.WriteLine(12345.ToStringWithSpaces());
Console.WriteLine(123456.ToStringWithSpaces());
Console.WriteLine(1234567.ToStringWithSpaces());
Console.WriteLine(32874123.ToStringWithSpaces());
Console.WriteLine(bigNumber.ToStringWithSpaces());
GetKeyFromUser("\nDone!! Press any key to exit...");
}
Output
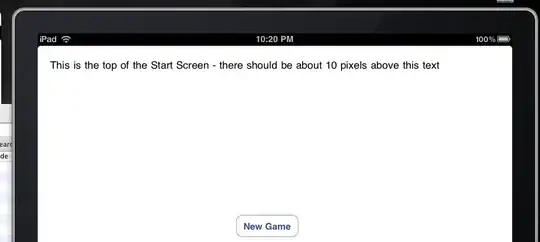
One nice thing about this approach is that you could also add the insertion position and separator string as arguments (with default values). This way, you could customize the output.
Here's a modified version:
public static class Extensions
{
public static string ToCustomString(this int number,
int position = 3, string separator = " ")
{
var numStr = number.ToString();
var len = numStr.Length;
var result = new StringBuilder();
for (var i = 0; i < len; i++)
{
if (i > 0 && i % position == 0) result.Insert(0, separator);
result.Insert(0, numStr[len - 1 - i]);
}
return result.ToString();
}
}
Now we can display the number with the default values (a space every three characters), or we could customize it. For example, if the number was being written in the middle of some text (or, even worse, if you were writing two numbers next to each other separated by a space), spaces might make it harder to read. In that case we might use dashes.
Here's an example:
private static void Main()
{
var bigNumber = int.MaxValue;
Console.WriteLine(bigNumber.ToString());
Console.WriteLine(bigNumber.ToCustomString());
Console.WriteLine(bigNumber.ToCustomString(2, "-"));
Console.WriteLine(bigNumber.ToCustomString(5, " < *_* > "));
GetKeyFromUser("\nDone!! Press any key to exit...");
}
Output
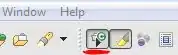