The simple way is setting QGraphicsColorizeEffect to the progress bar.
Like this:
QProgressBar* progress = new QProgressBar;
progress->setGraphicsEffect(new QGraphicsColorizeEffect);
Result on Win7:
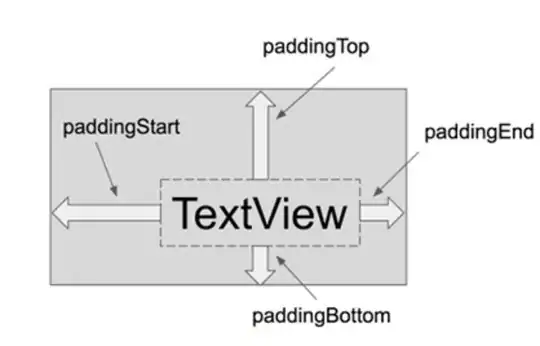
Umm...the result looks like fine, but we can make it better, only change the chunk colour.
Here is the final result:
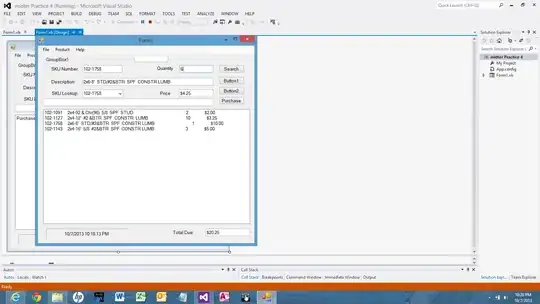
Reimplement QGraphicsEffect::draw
to specific & customize the colourize effect area:
class Colorize : public QGraphicsEffect {
public:
explicit Colorize(QObject *parent = Q_NULLPTR) :
QGraphicsEffect(parent),
strength(1),
color(Qt::red),
effectRect()
{ }
quint32 strength;
QColor color;
QRectF effectRect;
protected:
void draw(QPainter* painter) {
QPoint offset;
const QPixmap pixmap = sourcePixmap(Qt::LogicalCoordinates, &offset);
draw(painter, offset, pixmap, QRect());
}
void draw(QPainter *painter, const QPointF &dest, const QPixmap &src, const QRectF &srcRect) const
{
if (src.isNull())
return;
QImage srcImage;
QImage destImage;
if (srcRect.isNull()) {
srcImage = src.toImage();
srcImage = srcImage.convertToFormat(srcImage.hasAlphaChannel() ? QImage::Format_ARGB32_Premultiplied : QImage::Format_RGB32);
destImage = QImage(srcImage.size(), srcImage.format());
} else {
QRect rect = srcRect.toAlignedRect().intersected(src.rect());
srcImage = src.copy(rect).toImage();
srcImage = srcImage.convertToFormat(srcImage.hasAlphaChannel() ? QImage::Format_ARGB32_Premultiplied : QImage::Format_RGB32);
destImage = QImage(rect.size(), srcImage.format());
}
destImage.setDevicePixelRatio(src.devicePixelRatioF());
// do colorizing
QPainter destPainter(&destImage);
grayscale(srcImage, destImage, srcImage.rect());
destPainter.setCompositionMode(QPainter::CompositionMode_Screen);
destPainter.fillRect(effectRect, color);
destPainter.end();
// alpha blending srcImage and destImage
if(0 < strength && strength < 1){
QImage buffer = srcImage;
QPainter bufPainter(&buffer);
bufPainter.setOpacity(strength);
bufPainter.drawImage(0, 0, destImage);
bufPainter.end();
destImage = buffer;
}
if (srcImage.hasAlphaChannel())
destImage.setAlphaChannel(srcImage.alphaChannel());
painter->drawImage(dest, destImage);
}
};
Calculate the grove rect of the progress bar:
QRectF getGrooveRect() const {
StyleOptionProgressBar option;
option.initFrom(this); // this ⇒ progress bar
return style()->subElementRect(QStyle::SE_ProgressBarGroove, &option, this);
}
...
class StyleOptionProgressBar : public QStyleOptionProgressBar {
public:
using QStyleOptionProgressBar::QStyleOptionProgressBar;
void initFrom(const ColorizeProgressBar* w) {
init(w);
minimum = w->minimum();
maximum = w->maximum();
progress = w->value();
text = w->text();
textAlignment = w->alignment();
textVisible = w->isTextVisible();
orientation = w->orientation();
invertedAppearance = w->invertedAppearance();
}
};
Complete source on Github.
BTW, Referred Qt source code