Reference variables, not array variables
Your oldList
, list
, and newList
variables are all references, references that point to an array floating around in memory someplace. The references are not in themselves an array. Each of those three variables could be made to refer to a different array with a call such as oldList = { 97, 98 , 99 } ;
. Assigning a different array to a reference variable does not affect the content of the originally-assigned array.
In other words, oldList
, list
, and newList
are not “array variables”, they are “reference variables” that happen to point to some array or another.
Conceptually, you can think of it as shown in this diagram.
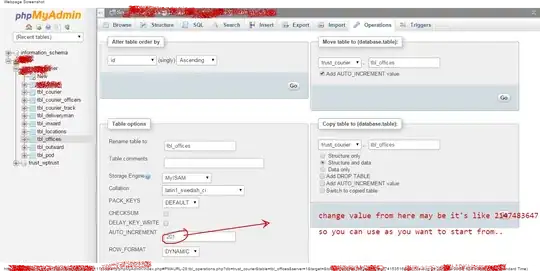
So your line:
list = newList;
…has no beneficial effect. It does indeed re-assign the argument reference variable list
from pointing to the blue first array to pointing to the salmon/yellow second array. But immediately after that re-assignment, the method ends. As the method ends, the list
variable goes out of scope, and disappears. That leaves the second array dangling out in memory in limbo, eventually to be garbage collected as no more references remain pointing to it. So no lasting effect. That re-assignment of list
has no effect on oldList
, contrary to your apparent expectation.
The upshot is that after calling reverse(oldList);
, you ended up with the same state as where you started, a reference variable oldList
pointing to the original array.
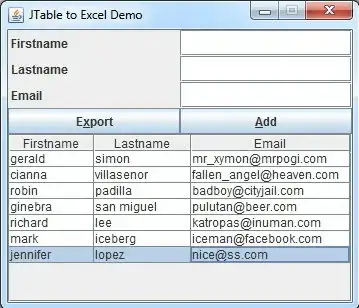
To get your desired results you could choose either of at least two other approaches:
- In the
reverse
method, make a new sorted array, and then when done, write those same sorted values back into the passed array. Let the new sorted array go out of scope when the method ends, to become a candidate for eventual garbage collection.
- Define the
reverse
method to return an array, rather than return void
. The calling method can then re-assign its own reference variable to point to the returned array.
oldList = reverse( oldList ) ;
I recommend the second approach, generally-speaking. The first alternative is usually a bad idea; messing around with passed values tends to lead to confusing logic. Usually better to give results back to the calling method, and let the calling method decide what to do with those results. This approach promotes loose coupling.
All of this is a fundamental piece of understanding Java and object-oriented programming. It will be tricky to grasp at first. Keep at it until it suddenly it snaps into place in your mind, then becoming second-nature, a barely conscious part of your thinking while programming.
By the way, while irrelevant to your direct question about learning the intricacies of Java and OOP, I want to mention that in practice for real-work I would accomplish your task with different code. I would use the Java Collections framework such as List
interface and ArrayList
concrete class rather than simple arrays, with Integer
class rather than int
primitive, assisted by auto-boxing and the new List.of
convenience method.
List < Integer > numbers = new ArrayList <>( List.of( 1 , 2 , 3 , 4 , 5 ) );
Collections.reverse( numbers );
The List.of
result is immutable, and cannot be re-ordered. So I feed its elements to an modifiable ArrayList
object’s constructor. The Collections.reverse
method modifies the ArrayList
, unlike with simple arrays.
Dump to console.
System.out.println( numbers );
[5, 4, 3, 2, 1]