Since you don't show your select
clause, I will do a sample select
; of course replace it with your own.
I have also created sample data, as you said you can't share yours, and stored in table1
, table2
and table3
:
var table1 = new List<Table1>()
{
new Table1() { ISIN = 1 },
new Table1() { ISIN = 4 }
};
var table2 = new List<Table2>() {
new Table2() { ISIN_DivReinv = 0, ISIN_GrowthDiv = 1, SchemeID = 111 },
new Table2() { ISIN_DivReinv = 1, ISIN_GrowthDiv = 0, SchemeID = 111 },
new Table2() { ISIN_DivReinv = 1, ISIN_GrowthDiv = 0, SchemeID = 222 },
new Table2() { ISIN_DivReinv = 2, ISIN_GrowthDiv = 2, SchemeID = 111 }
};
var table3 = new List<Table3>()
{
new Table3() { SchemeId = 111 },
new Table3() { SchemeId = 333 }
};
var Result =
from t1 in table1
from t2 in table2
where (t1.ISIN == t2.ISIN_GrowthDiv || t1.ISIN == t2.ISIN_DivReinv)
join t3 in table3 on t2.SchemeID equals t3.SchemeId
select new { t1.ISIN, t2.ISIN_DivReinv, t3.SchemeId };
These are the results I'm getting:
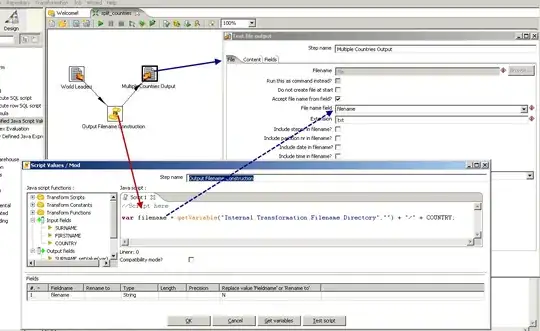
As you can see, there's no cross-join; which is not surprising, since by default LINQ provides inner joins with the join
operator and the only way to get cross joins is to just select without join, like in this answer.
EDIT: WHAT IF T3.SCHEMEID HAS DUPLICATES?
A "special" case is when t3
contains duplicated values, e.g. like
var table3 = new List<Table3>()
{
new Table3() { SchemeId = 111 },
new Table3() { SchemeId = 111 }
};
In this case you will get 4 rows instead of 2, since the inner join will properly occur for the two rows of t2
twice, one per matching value of t3
.
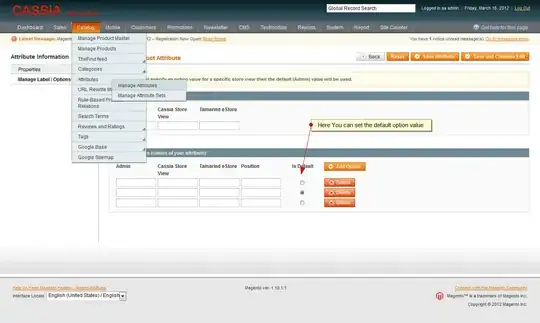
This doesn't make it a "cross-join" anyway, but you can take distinct values of t3.SchemeId if your select doesn't need values from t3
:
var Result =
from t1 in table1
from t2 in table2
from t3 in table3.Select(f => f.SchemeId).Distinct()
where (t1.ISIN == t2.ISIN_GrowthDiv || t1.ISIN == t2.ISIN_DivReinv) & t3 == t2.SchemeID
select new { t1.ISIN, t2.ISIN_DivReinv, t2.SchemeID };
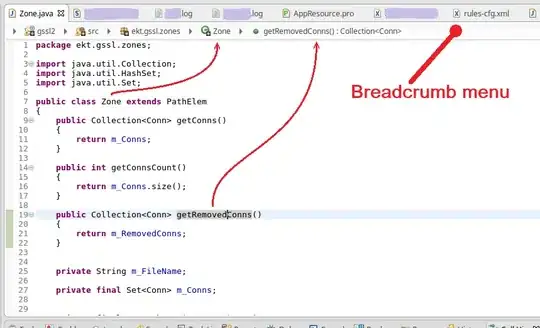