You cannot do this, without two loops. The reason being ng-content
is a placeholder, which accepts dynamic html inside. Now when you loop inside your code, you don't assign anything to the even
or odd
selector. But if you change your code to below
<child>
<div *ngFor="let number of numbers;let i = index" even>
<div *ngIf="(number%2 !== 0);else even">
<ul>{{number}}</ul>
</div>
<ng-template #even>
<ul>{{number}}</ul>
</ng-template>
<!-- <ul even>Why its rendering and not others??</ul> -->
</div>
</child>
You will see the output change to
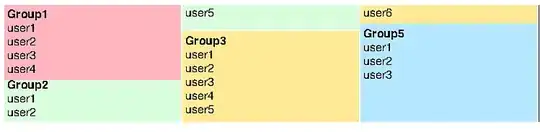
This is because no the output of ng-For
has been assigned to the even
selector.
If you change the template to below
<child>
<div *ngFor="let number of numbers;let i = index" odd>
<div *ngIf="(number%2 !== 0)">
<ul>{{number}}</ul>
</div>
</div>
<div *ngFor="let number of numbers;let i = index" even>
<div *ngIf="(number%2 == 0)">
<ul>{{number}}</ul>
</div>
</div>
</child>
It will work

Also remember inside a ng-For
you cannot append content to a even
or odd
section, even if that feature was available it would have overwritten the section with the last value.
Leaving even ng-for
out of it. The below code
<child>
<div>
<div odd>
This should be under odd
</div>
</div>
</child>
Also won't work

So what you are trying to achieve and the way you are trying to achieve it is not supported by angular.