Here's a working example using xlsx package :
totExpenses <- data.frame('Region'=c('Reg1','Reg2','Reg3'),
'Jan-18'=c(32,24,15),
'Feb-18'=c(65,45,78),
'Mar-18'=c(56,89,23),check.names=FALSE)
avgExpenses <- data.frame('Region'=c('Reg1','Reg2','Reg3'),
'Jan-18'=c(12,13,11),
'Feb-18'=c(14,15,14),
'Mar-18'=c(13,14,12),check.names=FALSE)
library(xlsx)
wb <- createWorkbook()
sh <- createSheet(wb,"mySheet")
row <- 1
addDataFrame(data.frame("Total Expenses"=double(),check.names=FALSE),
sheet = sh,startRow=row,row.names=FALSE)
row <- row + 2
addDataFrame(totExpenses,sheet=sh,startRow=row,row.names=FALSE)
row <- row + nrow(totExpenses) + 2
addDataFrame(data.frame("Average Expenses"=double(),check.names=FALSE),
sheet=sh,startRow=row,row.names=FALSE)
row <- row + 2
addDataFrame(avgExpenses,sheet=sh,startRow=row,row.names=FALSE)
saveWorkbook(wb,file='myfile.xlsx')
Result :
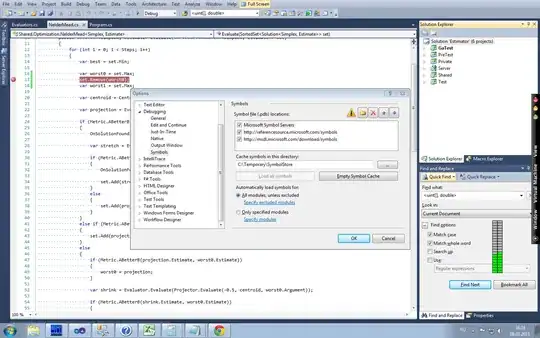
Here's a custom function that takes a named list of data.frame's and writes them to a excel sheet with the desired structure :
writeListOfDFToSheet <- function(lst,workBook,sheetName){
sh <- createSheet(workBook,sheetName)
# force names in list if not present
if(is.null(names(lst)))
names(lst) <- rep.int("",length(lst))
names(lst) <- ifelse(names(lst) == "",paste("data.frame",1:length(lst)),names(lst))
row <- 1
for(i in seq_len(length(lst))){
DFName <- names(lst)[i]
DF <- as.data.frame(lst[[i]])
# header
addDataFrame(setNames(data.frame(x=logical()),DFName),
sheet=sh,startRow=row,row.names=FALSE)
row <- row + 2
# data.frame
addDataFrame(DF,
sheet=sh,startRow=row,row.names=FALSE)
row <- row + nrow(DF) + 2
}
invisible(NULL)
}
Example of usage (assuming the previous 2 data.frame's being defined) :
# create a workbook
wb <- createWorkbook()
# write to "sheet 1"
lst1 <- list("Total Expenses"=totExpenses,"Average Expenses"=avgExpenses)
writeListOfDFToSheet(lst1,wb,"sheet 1")
# write to "sheet 2"
# (I'm using the same data.frame's but it's just an example)
lst2 <- list("Total Expenses"=totExpenses,"Average Expenses"=avgExpenses)
writeListOfDFToSheet(lst2,wb,"sheet 2")
# save excel file
saveWorkbook(wb,file='myfile.xlsx')