I don't see why you need two windows, so I'm going to assume you can get by with one window. You don't say so, but I'm assuming that a user will input the movie name, seat number, and row Id into text boxes on the window.
To answer your first question, all you have to do to bind a list to a DataGrid
is assign the list to the DataGrid
ItemsSource
property. For example (See the MainWindow
method below):
dataGridReservations.ItemsSource = reservations.List;
I'm new to WPF, but is seems that the default behavior for a DataGrid
is to create column names from the names of the variables in the list.
You want to implement your list of reservations as an ObservableCollection
because an ObservableCollection
automatically propagates changes to the datagrid when an item is added, deleted, or modified in the bound list. See How do I bind a List to a WPF DataGrid?
For your second question: Use the Add
button click event to add movie name, seat number, and row Id from the text boxes to a new item in the list. Again, when the list is updated, the DataGrid
is updated due to the action of the ObservableCollection
Here is the code that allows a user to input a movie name, seat number, and row id, and then click the Add button to add it to the DataGrid
. More code is needed to allow the user to edit or delete an item in the grid.
XAML follows the code
See screen shot at the bottom for a demo
public partial class MainWindow : Window
{
Reservations reservations;
public MainWindow()
{
InitializeComponent();
reservations = new Reservations();
dataGridReservations.ItemsSource = reservations.List;
}
public class Reservations
{
public class Reservation
{
private string _movieName;
private string _seat;
private string _rowID;
public Reservation(string movieName, string seat, string rowID)
{
MovieName = movieName;
Seat = seat;
RowID = rowID;
}
public string MovieName { get => _movieName; set => _movieName = value; }
public string Seat { get => _seat; set => _seat = value; }
public string RowID { get => _rowID; set => _rowID = value; }
}
private ObservableCollection<Reservation> _list;
public ObservableCollection<Reservation> List { get => _list; set => _list = value; }
public Reservations()
{
List = new ObservableCollection<Reservation>();
}
public void AddReservationToList(string MovieName, string SeatNumber, string RowId)
{
Reservation reservation = new Reservation(MovieName, SeatNumber, RowId);
List.Add(reservation);
}
}
private void ButtonAddReservationToList(object sender, RoutedEventArgs e)
{
reservations.AddReservationToList(textBoxMovieName.Text, textBoxSeatNumber.Text, textBoxRowId.Text);
textBoxMovieName.Text = "";
textBoxSeatNumber.Text = "";
textBoxRowId.Text = "";
}
}
MainWindow XAML
<Window x:Class="SO_Refresh_datagrid.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:SO_Refresh_datagrid"
mc:Ignorable="d"
Title="MainWindow" Height="350" Width="525">
<Grid>
<Button Content="Add" HorizontalAlignment="Left" Height="30" Margin="83,169,0,0" VerticalAlignment="Top" Width="64" Click="ButtonAddReservationToList"/>
<TextBox x:Name="textBoxMovieName" HorizontalAlignment="Left" Height="31" Margin="140,18,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="103"/>
<TextBox x:Name="textBoxSeatNumber" HorizontalAlignment="Left" Height="24" Margin="140,61,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="103"/>
<TextBox x:Name="textBoxRowId" HorizontalAlignment="Left" Height="24" Margin="140,100,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="103"/>
<Label Content="Movie Name" HorizontalAlignment="Left" Height="31" Margin="36,18,0,0" VerticalAlignment="Top" Width="71"/>
<Label Content="Seat Number" HorizontalAlignment="Left" Height="31" Margin="36,61,0,0" VerticalAlignment="Top" Width="90"/>
<Label Content="Row Id" HorizontalAlignment="Left" Height="31" Margin="36,100,0,0" VerticalAlignment="Top" Width="71"/>
<DataGrid x:Name="dataGridReservations" HorizontalAlignment="Left" Height="284" Margin="277,18,0,0" VerticalAlignment="Top" Width="209"/>
</Grid>
</Window>
Demo
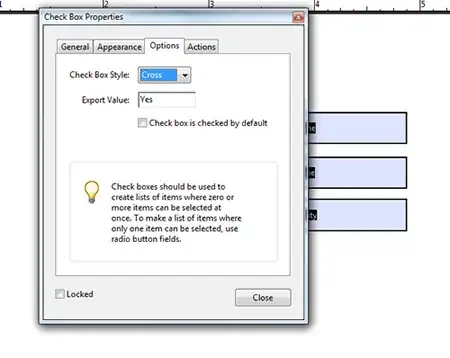