The circle()
method draws a circle, but the dot()
method stamps one out. However, the dot()
method doesn't have a separate line and fill concept and tends to overwrite itself, so we have to handle it carefully:
import turtle
def origin_circle(turtle, radius):
turtle.dot(radius + 2, 'black')
turtle.dot(radius, 'white')
for radius in range(200, 0, -40):
origin_circle(turtle, radius)
turtle.hideturtle()
turtle.mainloop()
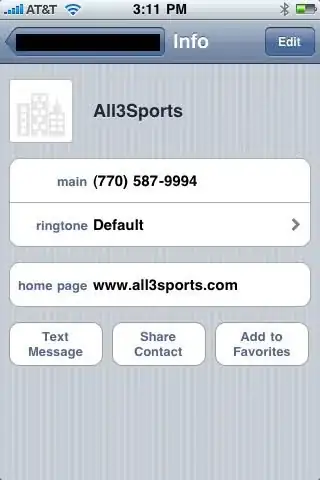
Alternatively, we could stamp out circular cursors ourselves:
import turtle
def origin_circle(turtle, radius):
turtle.shapesize(radius)
turtle.stamp()
turtle.shape('circle')
turtle.color('black', 'white')
for radius in range(10, 0, -2):
origin_circle(turtle, radius)
turtle.hideturtle()
turtle.mainloop()
But this doesn't produce as pleasing a result:
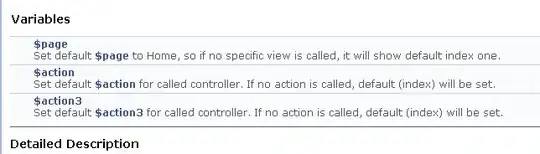
Of course, we can always cheat and use turtle.speed('fastest')
, or better yet, turn tracing off altogether:
import turtle
def origin_circle(turtle, radius):
turtle.penup()
turtle.sety(-radius)
turtle.pendown()
turtle.circle(radius, steps=90)
turtle.tracer(False)
for radius in range(20, 120, 20):
origin_circle(turtle, radius)
turtle.hideturtle()
turtle.tracer(True)
turtle.mainloop()
But the result still won't look as nice as the turtle.dot()
approach, even if you bump up the steps
parameter of turtle.circle()
:
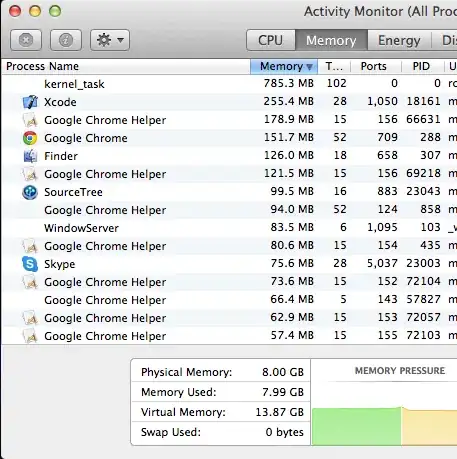
for the first code how to add an arrow on the top of each curve, one
at down of each curve?
This is easier done modifying my third example as we can more easily draw semicircles and stamp the cursor. I'm using a custom cursor for arrow alignment across circles purposes:
import turtle
def origin_circle(turtle, radius):
turtle.penup()
turtle.sety(-radius)
turtle.pendown()
turtle.stamp()
turtle.circle(radius, extent=180, steps=45)
turtle.stamp()
turtle.circle(radius, extent=180, steps=45)
turtle.addshape("pointer", ((0, 0), (5, -4), (0, 4), (-5, -4)))
turtle.shape("pointer")
turtle.tracer(False)
for idx, radius in enumerate(range(20, 120, 20), start=0):
origin_circle(turtle, radius)
turtle.hideturtle()
turtle.tracer(True)
turtle.mainloop()
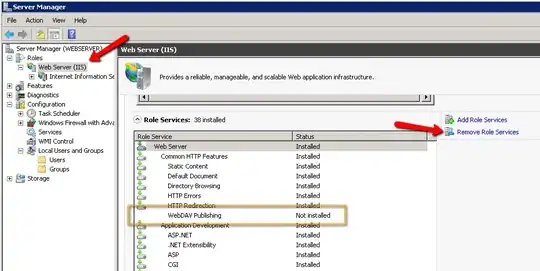