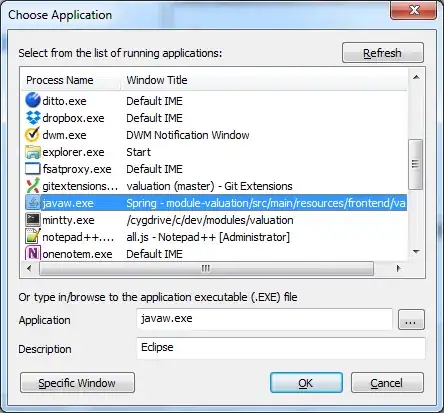
So this is what I have done in my one project , use FlexBoxLayout
, same in my case some pictures were very large in size , so had to do something about concurrency.
flexboxLayoutManager = new FlexboxLayoutManager(yourActivity.this);
flexboxLayoutManager.setFlexWrap(FlexWrap.WRAP);
flexboxLayoutManager.setAlignItems(AlignItems.STRETCH);
flexboxLayoutManager.setFlexDirection(FlexDirection.ROW);
recyclerView.setLayoutManager(flexboxLayoutManager);
Main logic comes in adapter, on onBindViewHolder
Glide.with(context)
.load(String.format("yourUrlHere")
.diskCacheStrategy(DiskCacheStrategy.ALL)
.animate(R.anim.fadein)
.override(dpToPx(90) ,dpToPx(90)) // here replace with your desired size.
.centerCrop()
.listener(new RequestListener<String, GlideDrawable>() {
@Override
public boolean onException(Exception e, String model, Target<GlideDrawable> target, boolean isFirstResource) {
// holder.loader.setVisibility(View.GONE);
return false;
}
@Override
public boolean onResourceReady(GlideDrawable resource, String model, Target<GlideDrawable> target, boolean isFromMemoryCache, boolean isFirstResource) {
// holder.loader.setVisibility(View.GONE);
return false;
}
})
.priority(Priority.IMMEDIATE)
.into(viewHolder.imageView);
ViewGroup.LayoutParams lp = viewHolder.imageView.getLayoutParams();
if (lp instanceof FlexboxLayoutManager.LayoutParams) {
FlexboxLayoutManager.LayoutParams flexboxLp = (FlexboxLayoutManager.LayoutParams) lp;
Random random = new Random();
int val = random.nextInt(5);
if (val ==0){
val = 1;
}
flexboxLp.setFlexGrow(val);
flexboxLp.setFlexShrink(1f);
}
And you can solve your problem , using this code, as i have implemented by myself in one project. Hope this solves your problem. Happy coding :)
Note : In my project depending upon requirement, I have used flexboxLayoutManager.setFlexDirection(FlexDirection.COLUMN);
so my layout is like this.