This is a bit of a workaround but you should have no problem implementing it in your case. I toyed around with it a bit and had better luck creating an actual fontface variable right in the dataset and using numerics, instead of c("plain", "bold")
.
library(tidyverse)
library(magrittr)
data("mtcars")
mtcars %<>%
rownames_to_column %>%
mutate(
rowname = rowname %>%
fct_reorder(mpg)
)
mtcars$font_face <- rep(c(1,2,3,4))
p1 <- mtcars %>%
ggplot(aes(mpg, rowname)) +
geom_point() +
theme(axis.text.y = element_text(face = mtcars$font_face)) +
facet_grid(cyl ~ .,
scales = "free_y",
space = "free_y")
p1
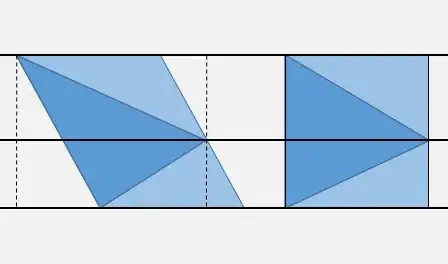
As you can see, the positions of the four faces are italic=1, bold=2, plain=3, and bold.italic=4.
So to recreate the example as you've presented, you just need to generate a variable in the original data piping and assign it using whatever relevant method. To recreate your example explicitly I'd go with the following code but I assume you'll want to work up a dplyr solution that actually fits your use case.
mtcars$font_face <- ifelse(mtcars$rowname %in% c("Toyota Corolla", "Fiat 128"), 2, 3)
edit:
The final solution is currently not working (the ifelse
line). It recognizes the else
value and selecting among 1:4
successfully changes the effect applied to the labels, but it doesn't pick change the labels for the if
values, despite the fact that the mtcars$font_face
variable does take on those values.
I'm stuck at the moment because I can't figure out why it ran for me before but not on a fresh session. I'm still working on figuring it out myself but perhaps my start can help someone to get to the next step.