To use my InputHelper library follow these steps:
Download the library (not the source code) from GitHub:
https://github.com/Visual-Vincent/InputHelper/releases
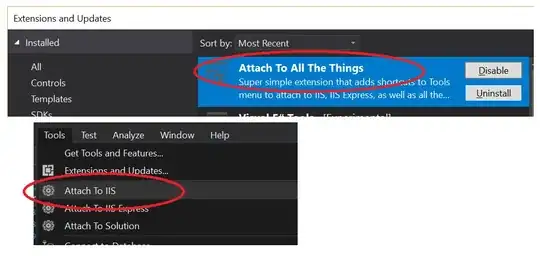
Extract the .zip
-file somewhere.
In Visual Studio, right-click your project in the Solution Explorer
and press Add Reference...
.
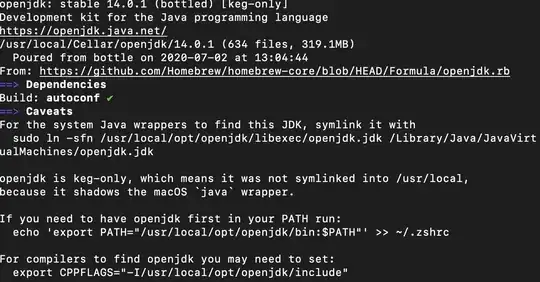
Go to the Browse
tab and locate the extracted folder from step 2, then select the InputHelper.dll
file.
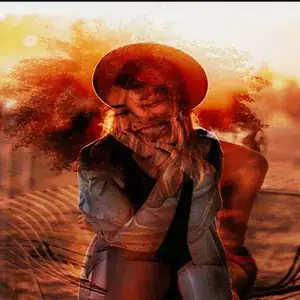
Now do step 3 again, but this time go to the .NET
tab and import System.Windows.Forms
(this is only needed if you are using a Console Application).
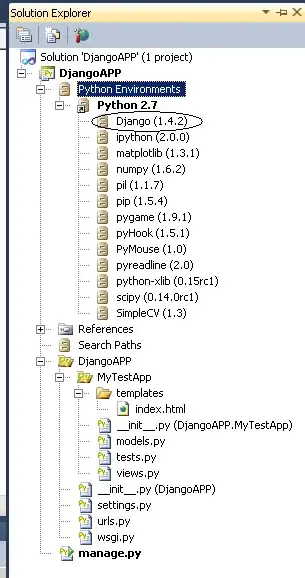
Finally, import the InputHelperLib
namespace as well as System.Windows.Forms
by writing this in the top of your code file:
Imports System.Windows.Forms
Imports InputHelperLib
Now you can use the InputHelper.Keyboard.PressKey()
method to programmatically press a key:
Imports System.Windows.Forms
Imports InputHelperLib
Module MainModule
Public Sub Main()
'Some code here...
InputHelper.Keyboard.PressKey(Keys.Enter)
'Some code here...
End Sub
End Module
KEEP IN MIND that if you are going to distribute this you must also include InputHelper's LICENSE.txt
file with every copy of your application.
You may rename the file as long as the user still understands what it is for.
InputHelper currently consists of four main categories:
InputHelper.Hooks
: Classes for capturing system-wide mouse and keyboard input. This can be used to make hot keys, for instance. See the project's wiki for implementation help.
InputHelper.Keyboard
: Methods for simulating physical keyboard input/key strokes.
InputHelper.Mouse
: Methods for simulating physical mouse input.
InputHelper.WindowMessages
: Methods for simulating mouse and keyboard input at a more virtual level. This utilizes window messages and can be used to target specific windows.
To explore the contents of my library right-click the namespace in your code and press Go To Definition
.
This will open the Object Browser
, in which you can browse the contents of my library, with all the types ("categories") on the left, and the methods/members on the right.