The following function draws an incomplete rectangle around the region of interest. I made use of cv2.line()
twice for each point provided. Additionally I also made use of cv2.circle()
to mark the 4 points.
There is one constraint. You will have to provide the 4 points in the following order: top-left, bottom-left, top-right, bottom-right.
Also there is an option to vary the length of the line you want to draw line_length
.
Code:
def draw_border(img, point1, point2, point3, point4, line_length):
x1, y1 = point1
x2, y2 = point2
x3, y3 = point3
x4, y4 = point4
cv2.circle(img, (x1, y1), 3, (255, 0, 255), -1) #-- top_left
cv2.circle(img, (x2, y2), 3, (255, 0, 255), -1) #-- bottom-left
cv2.circle(img, (x3, y3), 3, (255, 0, 255), -1) #-- top-right
cv2.circle(img, (x4, y4), 3, (255, 0, 255), -1) #-- bottom-right
cv2.line(img, (x1, y1), (x1 , y1 + line_length), (0, 255, 0), 2) #-- top-left
cv2.line(img, (x1, y1), (x1 + line_length , y1), (0, 255, 0), 2)
cv2.line(img, (x2, y2), (x2 , y2 - line_length), (0, 255, 0), 2) #-- bottom-left
cv2.line(img, (x2, y2), (x2 + line_length , y2), (0, 255, 0), 2)
cv2.line(img, (x3, y3), (x3 - line_length, y3), (0, 255, 0), 2) #-- top-right
cv2.line(img, (x3, y3), (x3, y3 + line_length), (0, 255, 0), 2)
cv2.line(img, (x4, y4), (x4 , y4 - line_length), (0, 255, 0), 2) #-- bottom-right
cv2.line(img, (x4, y4), (x4 - line_length , y4), (0, 255, 0), 2)
return img
line_length = 15
img = np.zeros((512,512,3), np.uint8)
cv2.imshow('img', img)
point1, point2, point3, point4 = (280,330), (280,390), (340,330), (340,390)
fin_img = draw_border(img, point1, point2, point3, point4, line_length)
cv2.imshow('fin_img', fin_img)
cv2.waitKey()
cv2.destroyAllWindows()
Result:
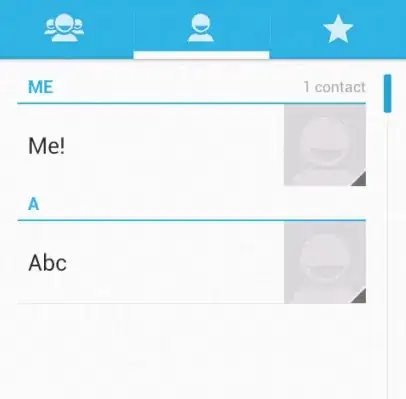