You may use one of the two approaches.
You may match all lines that start with //
and skip them, and only match your substrings in other contexts.
'~^(\s*//.*)(*SKIP)(*F)|^(?:\s*Connection\s+)?(.+?)\s*=\s*new\s+DBConnection~m'
See the regex demo
PHP demo:
$re = '~^(\s*//.*)(*SKIP)(*F)|^(?:\s*Connection\s+)?(.+?)\s*=\s*new\s+DBConnection~m';
$str = "Connection variable = new DBConnection\n variable = new DBConnection\n //\n //Connection variable = new DBConnection\n //variable = new DBConnection\n // Connection variable = new DBConnection\n // variable = new DBConnection";
if (preg_match_all($re, $str, $matches)) {
print_r($matches[0]);
}
Output:
Array
(
[0] => Connection variable = new DBConnection
[1] => variable = new DBConnection
)
Approach 2: Optional capturing group and a bit of post-processing
In PHP PCRE regex patterns, you cannot use infinite-width lookbehinds meaning the patterns inside cannot be quantified with *
, +
, *?
, +?
, ?
, ?
, {1,4}
, {3,}
quantifiers. Moreover, you cannot use nested alternation either.
A usual workaround is to use an optional capturing group and check its value after a match is found. If the group value is not empty, it means the match should be "failed", discarded, else, grab the capture you need.
Here is an example regex:
'~^(\s*//)?(?:\s*Connection\s+)?(.+?)\s*=\s*new\s+DBConnection~m'
See the regex demo:
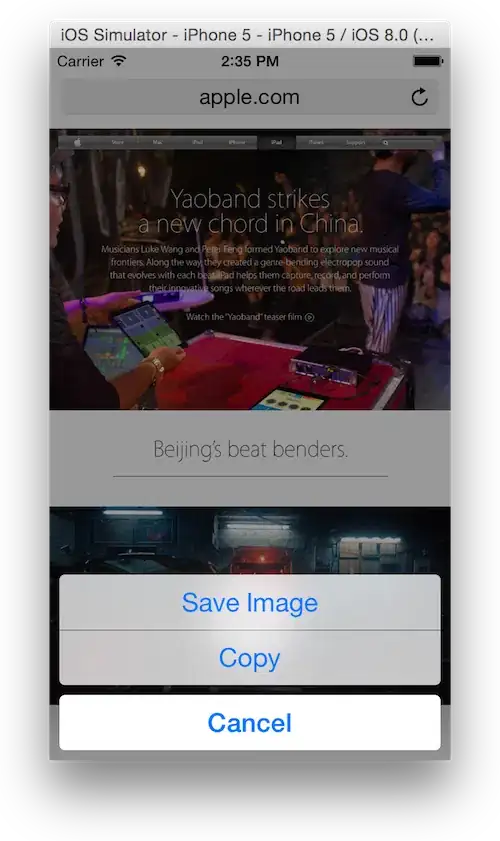
The green highlighted substrings are Group 1 matches. We can check them in the code like this:
$result = ""; // Result is empty
if (preg_match($rx, $s, $m)) { // Is there a match?
if (empty($m[1])) { // Is the match group #1 empty?
$result = $m[0]; // If yes, we found a result
}
} // Else, result will stay empty
See the PHP demo:
$strs = ['Connection variable = new DBConnection', 'variable = new DBConnection', '//Connection variable = new DBConnection', '//variable = new DBConnection'];
$rx = '~^(\s*//)?(?:\s*Connection\s+)?(.+?)\s*=\s*new\s+DBConnection~m';
foreach ($strs as $s) {
echo "$s:\n";
if (preg_match($rx, $s, $m)) {
if (empty($m[1])) {
echo "FOUND:" . $m[0] . "\n--------------\n";
}
} else {
echo "NOT FOUND\n--------------\n";
}
}
Output:
Connection variable = new DBConnection:
FOUND:Connection variable = new DBConnection
--------------
variable = new DBConnection:
FOUND:variable = new DBConnection
--------------
//Connection variable = new DBConnection:
//variable = new DBConnection:
Same technique can be used with preg_replace_callback
if you need to replace.